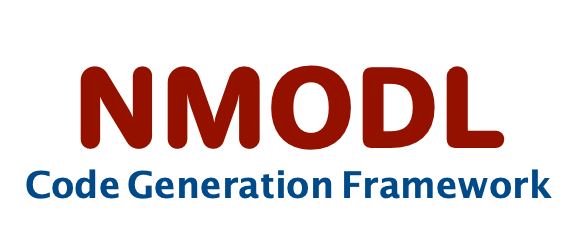 |
User Guide
|
Go to the documentation of this file.
68 CDriver(
bool strace,
bool ptrace);
71 static void error(
const std::string& m);
79 static void error(
const std::string& m,
const location& l);
89 bool is_typedef(
const std::string& type)
const noexcept {
98 const std::vector<std::string>&
all_tokens() const noexcept {
102 bool has_token(
const std::string& token)
const noexcept {
bool is_verbose() const noexcept
bool parse_string(const std::string &input)
parser c provided as string (used for testing)
bool is_enum_constant(const std::string &constant) const noexcept
encapsulates code generation backend implementations
bool parse_stream(std::istream &in)
parse c file provided as istream
void set_verbose(bool b) noexcept
Class that binds all pieces together for parsing C verbatim blocks.
std::vector< std::string > enum_constants
constants defined in enum
bool has_token(const std::string &token) const noexcept
bool parse_file(const std::string &filename)
std::string streamname
file or input stream name (used by scanner for position), see todo
const std::vector< std::string > & all_tokens() const noexcept
static void error(const std::string &m)
bool trace_scanner
enable debug output in the flex scanner
std::unique_ptr< CParser > parser
pointer to the parser instance being used
std::vector< std::string > tokens
all tokens encountered
bool trace_parser
enable debug output in the bison parser
std::map< std::string, std::string > typedefs
all typedefs
bool is_typedef(const std::string &type) const noexcept
std::unique_ptr< CLexer > lexer
pointer to the lexer instance being used
bool verbose
print messages from lexer/parser
void scan_string(const std::string &text)
void add_token(const std::string &)