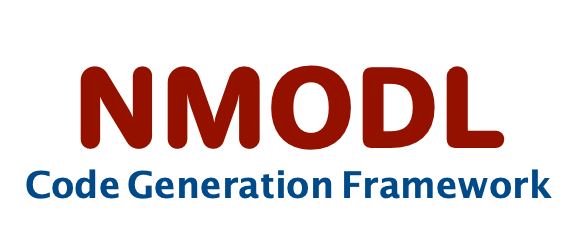 |
User Guide
|
Go to the documentation of this file.
19 #include <unordered_map>
20 #include <unordered_set>
175 return text == fmt::format(
"style_{}",
name);
179 return text == (
"di" +
name +
"dv");
215 return "di" +
name +
"dv";
226 auto ion =
Ion(ion_name);
227 return {ion.ionic_current_name(),
228 ion.intra_conc_name(),
229 ion.extra_conc_name(),
230 ion.rev_potential_name()};
261 throw std::runtime_error(fmt::format(
"Invalid `var_name == {}`.", var_name));
295 std::shared_ptr<ast::Expression>
rate_expr);
300 std::shared_ptr<ast::Expression>
volume(
const std::string& index_name)
const;
306 std::shared_ptr<ast::Expression>
diffusion_rate(
const std::string& index_name)
const;
309 double dfcdc(
const std::string& )
const;
313 const std::string& index_name,
314 const std::string& old_index_name,
315 const std::shared_ptr<ast::Expression>& old_expr)
const;
599 std::unordered_map<const ast::EigenNewtonSolverBlock*, std::string>
functor_names;
620 bool is_current(const std::
string& name) const noexcept;
std::vector< const ast::FunctionBlock * > functions
all functions defined in the mod file
std::shared_ptr< ast::Expression > volume(const std::string &index_name) const
Volume of this species.
bool emit_cvode
True if we have to emit CVODE code TODO: Figure out when this needs to be true.
double dfcdc(const std::string &) const
The value of what NEURON calls dfcdc.
int num_net_receive_parameters
number of arguments to net_receive block
std::string rate_index_name
std::string ion
name of the ion
bool ion_has_write_variable() const noexcept
if any ion has write variable
int tqitem_index
tqitem index in integer variables note that if tqitem doesn't exist then the default value should be ...
bool is_read(const std::string &name) const
std::shared_ptr< ast::Expression > rate_expr
std::vector< ast::Node * > top_verbatim_blocks
all top level verbatim blocks
bool for_netcon_used
if for_netcon is used
const ast::NetReceiveBlock * net_receive_node
net receive block for point process
std::vector< Ion > ions
ions used in the mod file
static std::vector< std::string > get_possible_variables(const std::string &ion_name)
for a given ion, return different variable names/properties like internal/external concentration,...
bool diam_used
if diam is used
bool thread_callback_register
if thread thread call back routines need to register
std::shared_ptr< ast::Expression > volume_expr
int watch_count
number of watch expressions
std::vector< const ast::DerivimplicitCallback * > derivimplicit_callbacks
derivimplicit callbacks need to be emited
bool is_rev_written() const
int derivimplicit_list_num
slist/dlist id for derivimplicit block
int thread_data_index
thread_data_index indicates number of threads being allocated.
bool derivimplicit_used() const
if legacy derivimplicit solver from coreneuron to be used
std::string rsuffix
point process range and functions don't have suffix
bool is_interior_conc_written() const
std::vector< SymbolType > table_statement_variables
table variables
const ast::InitialBlock * initial_node
initial block
int num_primes
number of primes (all state variables not necessary to be prime)
std::vector< SymbolType > table_assigned_variables
bool net_send_used
if net_send function is used
std::optional< double > valence
ion valence
bool is_ionic_variable(const std::string &text) const
Is the variable name text related to this ion?
encapsulates code generation backend implementations
Represent ions used in mod file.
Represents a block used for variable timestep integration (CVODE) of DERIVATIVE blocks.
Implement classes for representing symbol table at block and file scope.
const ast::ConstructorBlock * constructor_node
constructor block
std::string extra_conc_name() const
int top_local_thread_size
total length of all top local variables
std::string name
name of the ion
bool is_voltage_used_by_watch_statements() const
true if WatchStatement uses voltage v variable
std::string changed_dt
updated dt to use with steadystate solver (in initial block) empty string means no change in dt
bool is_style(const std::string &text) const
Is the variable name text the style of this ion?
std::vector< SymbolType > constant_variables
constant variables
std::string rev_potential_name() const
std::unordered_map< const ast::EigenNewtonSolverBlock *, std::string > functor_names
unique functor names for all the EigenNewtonSolverBlock s
bool bbcore_pointer_used
if bbcore pointer is used
std::string mod_suffix
name of the suffix
std::vector< const ast::ProcedureBlock * > procedures
all procedures defined in the mod file
Represent information collected from AST for code generation.
std::vector< SymbolType > global_variables
global variables
std::shared_ptr< ast::Expression > substitute_index(const std::string &index_name, const std::string &old_index_name, const std::shared_ptr< ast::Expression > &old_expr) const
std::string extra_conc_pointer_name() const
std::string rev_potential_pointer_name() const
int table_count
number of table statements
int first_pointer_var_index
index/offset for first pointer variable if exist
bool thread_safe
if mod file is thread safe (always true for coreneuron)
std::string intra_conc_name() const
std::vector< std::string > writes
ion variables that are being written
std::vector< SymbolType > state_vars
all state variables
std::vector< const ast::WatchStatement * > watch_statements
all watch statements
int semantic_variable_count
number of semantic variables
int thread_var_data_size
sum of length of thread promoted variables
Represents a INITIAL block in the NMODL.
std::vector< IndexSemantics > semantics
index variable semantic information
bool artificial_cell
if mod file is artificial cell
bool area_used
if area is used
bool is_rev_potential(const std::string &text) const
Check if variable name is reveral potential.
const ast::CvodeBlock * cvode_block
the CVODE block
IndexSemantics(int index, std::string name, int size)
std::string name
name/type of the variable (i.e. semantics)
Represents a BREAKPOINT block in NMODL.
std::vector< SymbolType > top_local_variables
local variables in the global scope
bool need_style
if style semantic needed
int num_solve_blocks
number of solve blocks in mod file
Represents a DESTRUCTOR block in the NMODL.
bool declared_thread_safe
A mod file can be declared to be thread safe using the keyword THREADSAFE.
bool eigen_linear_solver_exist
true if eigen linear solver is used
std::vector< SymbolType > thread_variables
thread variables (e.g. global variables promoted to thread)
Represents a CONSTRUCTOR block in the NMODL.
std::vector< ast::Node * > top_blocks
all top level global blocks
bool is_ionic_current(const std::string &name) const noexcept
if given variable is a ionic current
std::string current_derivative_pointer_name() const
std::unordered_set< std::string > variables_in_verbatim
all variables/symbols used in the verbatim block
bool require_wrote_conc
if we need a call back to wrote_conc in neuron/coreneuron
bool is_exterior_conc_written() const
Represent semantic information for index variable.
bool is_intra_cell_conc(const std::string &text) const
Check if variable name is internal cell concentration.
int size
number of elements (typically one)
const ast::BreakpointBlock * breakpoint_node
derivative block
bool eigen_newton_solver_exist
true if eigen newton solver is used
bool is_extra_cell_conc(const std::string &text) const
Check if variable name is external cell concentration.
std::vector< std::string > currents
non specific and ionic currents
bool is_conc_read() const
Information required to print LONGITUDINAL_DIFFUSION callbacks.
int first_random_var_index
index/offset for first RANDOM variable if exist
bool point_process
if mod file is point process
std::string ionic_current_pointer_name() const
const ast::NrnStateBlock * nrn_state_block
nrn_state block
bool vectorize
true if mod file is vectorizable (which should be always true for coreneuron) But there are some bloc...
std::map< std::string, LongitudinalDiffusionInfo > longitudinal_diffusion_info
for each state, the information needed to print the callbacks.
std::string variable
ion variable like intra/extra concentration
std::string mod_file
name of mod file
std::vector< SymbolType > range_assigned_vars
range variables which are assigned variables as well
std::vector< const ast::Block * > functions_with_table
function or procedures with table statement
bool is_conc_written() const
bool is_ionic_conc(const std::string &text) const
check if it is either internal or external concentration
bool is_ion_write_variable(const std::string &name) const noexcept
if given variable is ion write variable
std::vector< const ast::FunctionTableBlock * > function_tables
all functions tables defined in the mod file
int primes_size
sum of length of all prime variables
Auto generated AST classes declaration.
bool function_uses_table(const std::string &name) const noexcept
std::shared_ptr< ast::Expression > diffusion_rate(const std::string &index_name) const
Difusion rate of this species.
bool net_event_used
if net_event function is used
int variable_index(const std::string &var_name) const
Variable index in the ion mechanism.
int derivimplicit_var_thread_id
thread id for derivimplicit variables
Represents the coreneuron nrn_state callback function.
std::vector< Conductance > conductances
represent conductance statements used in mod file
int index
start position in the int array
bool emit_table_thread() const noexcept
std::vector< std::pair< SymbolType, std::string > > neuron_global_variables
[Core]NEURON global variables used (e.g. celsius) and their types
bool is_written(const std::string &name) const
bool electrode_current
if electrode current specified
std::string current_derivative_name() const
bool is_current_derivative(const std::string &text) const
std::vector< SymbolType > external_variables
external variables
bool is_interior_conc_read() const
std::vector< std::string > implicit_reads
ion variables that are being implicitly read
const ast::InitialBlock * net_receive_initial_node
initial block within net receive block
bool is_ion_read_variable(const std::string &name) const noexcept
if given variable is ion read variable
bool is_exterior_conc_read() const
std::string volume_index_name
std::vector< const ast::FactorDef * > factor_definitions
all factors defined in the mod file
std::vector< SymbolType > prime_variables_by_order
this is the order in which they appear in derivative block this is required while printing them in in...
std::vector< SymbolType > pointer_variables
pointer or bbcore pointer variables
bool is_watch_used() const noexcept
if watch statements are used
int thread_var_thread_id
thread id for thread promoted variables
bool nrn_state_has_eigen_solver_block() const
true if EigenNewtonSolver is used in nrn_state block
std::vector< SymbolType > range_parameter_vars
range variables which are parameter as well
int top_local_thread_id
Top local variables are those local variables that appear in global scope.
static bool ends_with(const std::string &haystack, const std::string &needle)
Check if haystack ends with needle.
Represent conductance statements used in mod file.
std::shared_ptr< symtab::Symbol > SymbolType
const ast::DestructorBlock * destructor_node
destructor block only for point process
std::vector< const ast::Block * > before_after_blocks
all before after blocks
bool is_ionic_conc(const std::string &name) const noexcept
if given variable is a ionic concentration
int num_equations
number of equations (i.e.
bool is_ion_variable(const std::string &name) const noexcept
if either read or write variable
std::vector< SymbolType > use_ion_variables
new one used in print_ion_types
bool is_current(const std::string &name) const noexcept
if given variable is a current
std::vector< SymbolType > assigned_vars
remaining assigned variables
std::string ionic_current_name() const
bool write_concentration
if write concentration call required in initial block
LongitudinalDiffusionInfo(const std::shared_ptr< ast::Name > &index_name, std::shared_ptr< ast::Expression > volume_expr, const std::shared_ptr< ast::Name > &rate_index_name, std::shared_ptr< ast::Expression > rate_expr)
std::vector< SymbolType > random_variables
RANDOM variables.
std::vector< std::string > reads
ion variables that are being read
std::vector< SymbolType > range_state_vars
state variables excluding such useion read/write variables that are not ionic currents.
bool is_ionic_current(const std::string &text) const
Check if variable name is a ionic current.
std::string intra_conc_pointer_name() const
static constexpr char ION_VARNAME_PREFIX[]
prefix for ion variable