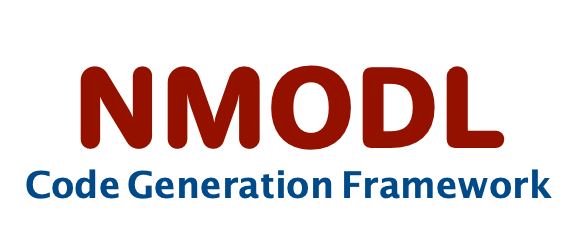 |
User Guide
|
Go to the documentation of this file.
56 if (
const char* nmodl_home = std::getenv(
"NMODLHOME")) {
57 auto path = std::string(nmodl_home) +
"/share/nmodl/nrnunits.lib";
63 std::ifstream f(path.c_str());
68 std::ostringstream err_msg;
69 err_msg <<
"Could not find nrnunits.lib in any of:\n";
71 err_msg << path <<
"\n";
73 throw std::runtime_error(err_msg.str());
encapsulates code generation backend implementations
Project version information.
static std::vector< std::string > NRNUNITSLIB_PATH
paths where nrnunits.lib can be found
static const std::string GIT_REVISION
git revision id
static const std::string SHARED_LIBRARY_SUFFIX
Information of units database i.e.
static std::string to_string()
return version string (version + git id) as a string
static const std::string NMODL_VERSION
project tagged version in the cmake
static std::string get_path()
Return path of units database file.
Common utility functions for file/dir manipulation.