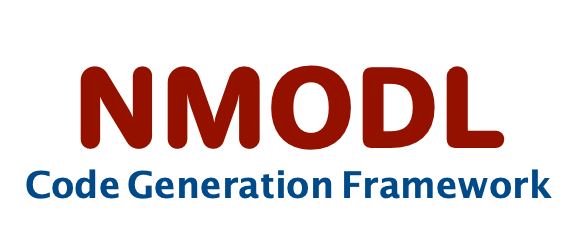 |
User Guide
|
Go to the documentation of this file.
55 static std::string
solve(
const std::string& equation, std::string method,
bool debug =
false);
58 static bool cnexp_possible(
const std::string& equation, std::string& solution);
static bool cnexp_possible(const std::string &equation, std::string &solution)
check if given equation can be solved using cnexp method
encapsulates code generation backend implementations
static std::string solve_equation(std::string &state, int order, std::string &rhs, std::string &method, bool &cnexp_possible, bool debug=false)
static void parse_equation(const std::string &equation, std::string &state, std::string &rhs, int &order)
parse given equation into lhs, rhs and find it's order and state variable
Class that binds all pieces together for parsing differential equations.
static std::string solve(const std::string &equation, std::string method, bool debug=false)
solve equation using provided method