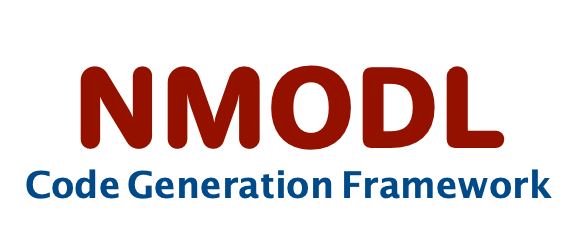 |
User Guide
|
Go to the documentation of this file.
15 Enum type for binary operators in NMODL
17 NMODL support different binary operators and this
18 type is used to store their value in the AST. See
19 nmodl::ast::Ast for details.
25 Enum type for every AST node type
27 Every node in the ast has associated type represented by
28 this enum class. See nmodl::ast::AstNodeType for details.
35 Base class for all Abstract Syntax Tree node types
37 Every node in the Abstract Syntax Tree is inherited from base class
38 Ast. This class provides base properties and functions that are implemented
45 Accept (or visit) the current AST node using current visitor
47 Instead of visiting children of AST node, like Ast::visit_children,
48 accept allows to visit the current node itself using the concrete
52 v (Visitor): Concrete visitor that will be used to recursively visit node
58 Visit children i.e. member of current AST node using provided visitor
60 Different nodes in the AST have different members (i.e. children). This method
61 recursively visits children using provided concrete visitor.
64 v (Visitor): Concrete visitor that will be used to recursively visit node
70 Return type (ast.AstNodeType) of the ast node
76 Return type (ast.AstNodeType) of the ast node as string
82 Return name of the node
84 Some ast nodes have a member designated as node name. For example,
85 in case of ast.FunctionCall, name of the function is returned as a node
86 name. Note that this is different from ast node type name and not every
93 Return nmodl statement of the node
95 Some ast nodes have a member designated as nmodl name. For example,
96 in case of "NEURON { }" the statement of NMODL which is stored as nmodl
97 name is "NEURON". This function is only implemented by node types that
98 have a nmodl statement.
105 Create a copy of the AST node
111 Return associated token for the AST node
117 Return associated symbol table for the AST node
119 Certain ast nodes (e.g. inherited from ast.Block) have associated
120 symbol table. These nodes have nmodl.symtab.SymbolTable as member
121 and it can be accessed using this method.
127 Return associated statement block for the AST node
129 Top level block nodes encloses all statements in the ast::StatementBlock.
130 For example, ast.BreakpointBlock has all statements in curly brace (`{ }`)
131 stored in ast.StatementBlock :
134 SOLVE states METHOD cnexp
135 gNaTs2_t = gNaTs2_tbar*m*m*m*h
136 ina = gNaTs2_t*(v-ena)
139 This method return enclosing statement block.
145 Negate the value of AST node
151 Set name for the AST node
157 Check if current node is of type ast.Ast
163 Return value of the ast node
169 Get or set the parent of this node
constexpr const char * set_name_method()
constexpr const char * get_node_type_method()
constexpr const char * accept_method()
constexpr const char * clone_method()
constexpr const char * get_node_type_name_method()
constexpr const char * parent_property()
constexpr const char * negate_method()
encapsulates code generation backend implementations
constexpr const char * is_ast_method()
constexpr const char * get_token_method()
constexpr const char * ast_nodetype_enum()
constexpr const char * get_node_name_method()
constexpr const char * eval_method()
constexpr const char * get_nmodl_name_method()
constexpr const char * get_symbol_table_method()
constexpr const char * get_statement_block_method()
constexpr const char * visit_children_method()
constexpr const char * ast_class()
constexpr const char * binary_op_enum()