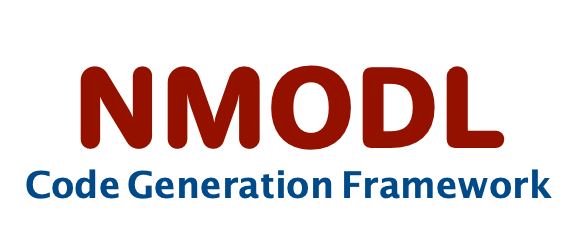 |
User Guide
|
Go to the documentation of this file.
16 namespace fs = std::filesystem;
29 const auto value = getenv(env_var.c_str());
30 if (value !=
nullptr) {
55 if (file.is_absolute() && fs::exists(file)) {
58 for (
auto paths_it =
paths_.rbegin(); paths_it !=
paths_.rend(); ++paths_it) {
59 auto file_abs = *paths_it / file;
60 if (fs::exists(file_abs)) {
61 return paths_it->string();
void append_env_var(const std::string &env_var)
std::string find_file(const std::filesystem::path &file)
Search a file.
void push_current_directory(const std::filesystem::path &path)
std::vector< std::filesystem::path > paths_
inclusion path list
encapsulates code generation backend implementations
Implement string manipulation functions.
void pop_current_directory()
static std::vector< std::string > split_string(const std::string &text, char delimiter)
Split a text in a list of words, using a given delimiter character.
static FileLibrary default_instance()
Initialize the library with the following path:
void push_cwd()
push the working directory in the directories stack
static constexpr char envpathsep
The character conventionally used by the operating system to separate search path components.
Common utility functions for file/dir manipulation.