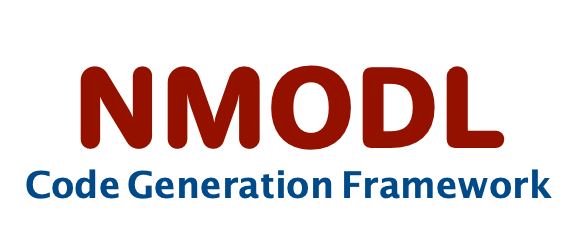 |
User Guide
|
Go to the documentation of this file.
44 const std::shared_ptr<ast::Expression>& node)
const;
std::string index
index variable name
bool under_indexed_name
true if we are visiting index variable
encapsulates code generation backend implementations
Helper visitor to replace index of array variable with integer.
Represents specific element of an array variable.
Concrete visitor for all AST classes.
void visit_binary_expression(ast::BinaryExpression &node) override
visit node of type ast::BinaryExpression
int value
integer value of index variable
void visit_indexed_name(ast::IndexedName &node) override
visit node of type ast::IndexedName
std::shared_ptr< ast::Expression > replace_for_name(const std::shared_ptr< ast::Expression > &node) const
if expression we are visiting is Name then return new Integer node
Represents binary expression in the NMODL.
Auto generated AST classes declaration.
IndexRemover(std::string index, int value)
Concrete visitor for all AST classes.