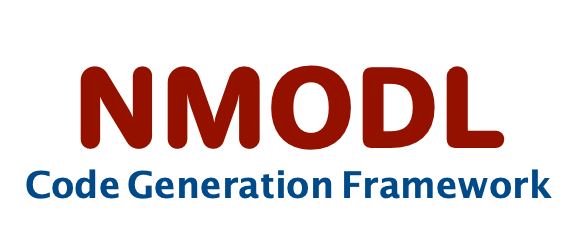 |
User Guide
|
const std::map< std::string, NmodlTestCase > nmodl_valid_constructs
encapsulates code generation backend implementations
std::string name
name of the test
std::string method
solve method
std::string name
name of the mod file
const std::vector< DiffEqTestCase > diff_eq_constructs
const std::map< std::string, NmodlTestCase > nmodl_invalid_constructs
Guidelines for adding nmodl text constructs.
std::string output
expected nmodl output
represent nmodl test construct
NmodlTestCase(std::string name, std::string input, std::string output)
std::string equation
differential equation to solve
represent differential equation test construct
std::string solution
expected solution
NmodlTestCase(std::string name, std::string input)
std::string input
input nmodl construct