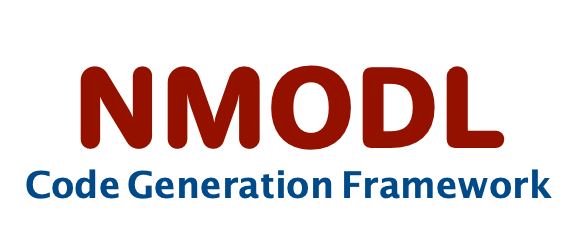 |
User Guide
|
Go to the documentation of this file.
20 #include <unordered_map>
21 #include <unordered_set>
223 const std::vector<std::string>& solutions,
227 const std::string& tmp_unique_prefix);
255 std::shared_ptr<ast::Expression> get_lhs(
const ast::Node& node));
279 inline size_t n()
const {
316 StatementDispenser(
const std::vector<std::string>::const_iterator& statements_str_beg,
317 const std::vector<std::string>::const_iterator& statements_str_end,
338 const std::string& var);
344 const size_t n_next_statements);
const std::unordered_set< ast::Statement * > * to_be_removed
group of old statements that need replacing
Base class for all AST node.
StatementDispenser pre_solve_statements
Update state variable statements (i.e. )
void try_replace_tagged_statement(const ast::Node &node, std::shared_ptr< ast::Expression > get_lhs(const ast::Node &node))
Try to replace a statement.
Represents differential equation in DERIVATIVE block.
int replaced_statements_end() const
idx (in the new statementVector) of the last statement that was added.
Replace statements in node with pre_solve_statements, tmp_statements, and solutions.
void tag_all_statements()
Mark that all the statements need updating (probably unused)
int error_on_n_flushes
Emit error when n_flushes reaches this number. -1 disables the error entirely.
One equation in a system of equations that collectively make a NONLINEAR block.
void build_maps()
Construct the maps var2dependants, var2statement and dependency_map for easy access and classificatio...
int replaced_statements_begin() const
idx (in the new statementVector) of the first statement that was added.
StatementDispenser()=default
Empty ctor.
std::vector< std::shared_ptr< Statement > > StatementVector
void visit_statement_block(ast::StatementBlock &node) override
visit node of type ast::StatementBlock
size_t n_interleaves
Number of interleaves of assignment statements in between equations of the system of equations.
encapsulates code generation backend implementations
std::set< size_t > tags
Keeps track of what statements need updating.
std::pair< int, int > replaced_statements_range
{begin index, end index} of the added statements. -1 means that it is invalid
StatementDispenser tmp_statements
tmp statements that appear with –cse (i.e. )
std::unordered_map< std::string, size_t > var2statement
a (key) : a = f(...) (value)
void visit_lin_equation(ast::LinEquation &node) override
visit node of type ast::LinEquation
Sorts and maps statements to variables keeping track of what needs updating.
Count interleaves of assignment statement inside the system of equations.
std::unordered_map< std::string, std::set< size_t > > var2dependants
a (key) : f(..., a, ...), g(..., a, ...), h(..., a, ...), ...
void new_equation(const bool is_in_system)
Count interleaves defined as a switch false -> true for in_system.
void visit_binary_expression(ast::BinaryExpression &node) override
visit node of type ast::BinaryExpression
void visit_diff_eq_expression(ast::DiffEqExpression &node) override
visit node of type ast::DiffEqExpression
ReplacePolicy policy
Replacement policy used by the various visitors.
Concrete visitor for all AST classes.
InterleavesCounter interleaves_counter
counts how many times the solution statements are interleaved with assignment expressions
size_t n_flushes
Max number of times a statement was printed using an emplace_back_all_tagged_statements command.
size_t tag_dependant_statements(const std::string &var)
Tag all the statements that depend on var for updating.
@ GREEDY
Replace statements greedily.
SympyReplaceSolutionsVisitor()=delete
Empty ctor.
std::unordered_map< std::shared_ptr< ast::Statement >, ast::StatementVector > replacements
Replacements found by the visitor.
StatementDispenser solution_statements
solutions that we want to replace
std::vector< std::shared_ptr< ast::Statement > > statements
Vector of statements.
Represents block encapsulating list of statements.
size_t emplace_back_all_tagged_statements(ast::StatementVector &new_statements)
Emplace back all the statements that are marked for updating in tags.
One equation in a system of equations tha collectively form a LINEAR block.
bool try_emplace_back_tagged_statement(ast::StatementVector &new_statements, const std::string &var)
Look for var in var2statement and emplace back that statement in new_statements.
bool is_var_assigned_here(const std::string &var) const
Check if one of the statements assigns this variable (i.e.
bool is_top_level_statement_block
Used to notify to visit_statement_block was called by the user (or another visitor) or re-called in a...
size_t n_next_equations
Number of solutions that match each old_statement with the greedy policy.
size_t emplace_back_next_tagged_statements(ast::StatementVector &new_statements, const size_t n_next_statements)
Emplace back the next n_next_statements solutions in statements that is marked for updating in tags.
Represents binary expression in the NMODL.
@ VALUE
Replace statements matching by lhs varName.
std::unordered_map< std::string, std::unordered_set< std::string > > dependency_map
x (key) : f(a, b, c, ...) (values)
void visit_non_lin_equation(ast::NonLinEquation &node) override
visit node of type ast::NonLinEquation
bool in_system
Bool that keeps track if just wrote an equation of the system of equations (true) or not (false)
size_t n() const
Number of interleaves.
Concrete visitor for all AST classes.