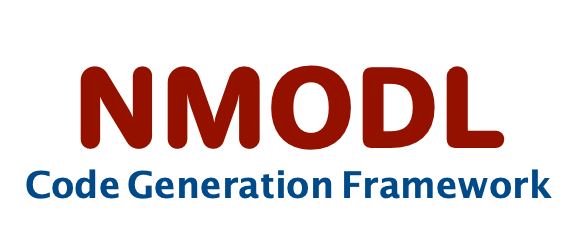 |
User Guide
|
Go to the documentation of this file. 1 #include <catch2/catch_test_macros.hpp>
2 #include <catch2/matchers/catch_matchers_string.hpp>
9 SECTION(
"empty substring") {
13 SECTION(
"empty str") {
17 SECTION(
"both empty") {
28 SECTION(
"oversized") {
34 SECTION(
"empty substring") {
38 SECTION(
"empty str") {
42 SECTION(
"both empty") {
53 SECTION(
"oversized") {
59 SECTION(
"both empty") {
63 SECTION(
"lhs emtpy") {
67 SECTION(
"rhs empty") {
71 SECTION(
"neither empty") {
static bool starts_with(const std::string &haystack, const std::string &needle)
Check if haystack starts with needle.
std::string join_arguments(const std::string &lhs, const std::string &rhs)
Joint two (list of) arguments.
encapsulates code generation backend implementations
Implement string manipulation functions.
static bool ends_with(const std::string &haystack, const std::string &needle)
Check if haystack ends with needle.