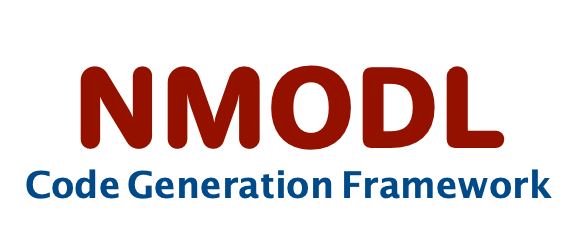 |
User Guide
|
Go to the documentation of this file.
15 #include "parser/nmodl/nmodl_parser.hpp"
19 using Token = parser::NmodlParser::token;
36 const static std::map<std::string, TokenType>
keywords = {
37 {
"VERBATIM", Token::VERBATIM},
38 {
"COMMENT", Token::BLOCK_COMMENT},
39 {
"TITLE", Token::MODEL},
40 {
"CONSTANT", Token::CONSTANT},
41 {
"PARAMETER", Token::PARAMETER},
42 {
"INDEPENDENT", Token::INDEPENDENT},
43 {
"ASSIGNED", Token::ASSIGNED},
44 {
"INITIAL", Token::INITIAL1},
45 {
"DERIVATIVE", Token::DERIVATIVE},
46 {
"EQUATION", Token::BREAKPOINT},
47 {
"BREAKPOINT", Token::BREAKPOINT},
48 {
"CONDUCTANCE", Token::CONDUCTANCE},
49 {
"SOLVE", Token::SOLVE},
50 {
"STATE", Token::STATE},
51 {
"LINEAR", Token::LINEAR},
52 {
"NONLINEAR", Token::NONLINEAR},
53 {
"DISCRETE", Token::DISCRETE},
54 {
"FUNCTION", Token::FUNCTION1},
55 {
"FUNCTION_TABLE", Token::FUNCTION_TABLE},
56 {
"PROCEDURE", Token::PROCEDURE},
57 {
"DEL2", Token::DEL2},
59 {
"LOCAL", Token::LOCAL},
60 {
"METHOD", Token::USING},
61 {
"STEADYSTATE", Token::STEADYSTATE},
62 {
"STEP", Token::STEP},
63 {
"WITH", Token::WITH},
64 {
"FROM", Token::FROM},
68 {
"else", Token::ELSE},
69 {
"while", Token::WHILE},
70 {
"START", Token::START1},
71 {
"DEFINE", Token::DEFINE1},
72 {
"KINETIC", Token::KINETIC},
73 {
"CONSERVE", Token::CONSERVE},
76 {
"SWEEP", Token::SWEEP},
77 {
"COMPARTMENT", Token::COMPARTMENT},
78 {
"LONGITUDINAL_DIFFUSION", Token::LONGDIFUS},
79 {
"SOLVEFOR", Token::SOLVEFOR},
80 {
"UNITS", Token::UNITS},
81 {
"UNITSON", Token::UNITSON},
82 {
"UNITSOFF", Token::UNITSOFF},
83 {
"TABLE", Token::TABLE},
84 {
"DEPEND", Token::DEPEND},
85 {
"NEURON", Token::NEURON},
86 {
"SUFFIX", Token::SUFFIX},
87 {
"POINT_PROCESS", Token::SUFFIX},
88 {
"ARTIFICIAL_CELL", Token::SUFFIX},
89 {
"NONSPECIFIC_CURRENT", Token::NONSPECIFIC},
90 {
"ELECTRODE_CURRENT", Token::ELECTRODE_CURRENT},
91 {
"RANGE", Token::RANGE},
92 {
"USEION", Token::USEION},
93 {
"READ", Token::READ},
94 {
"REPRESENTS", Token::REPRESENTS},
95 {
"WRITE", Token::WRITE},
96 {
"VALENCE", Token::VALENCE},
97 {
"CHARGE", Token::VALENCE},
98 {
"GLOBAL", Token::GLOBAL},
99 {
"POINTER", Token::POINTER},
100 {
"RANDOM", Token::RANDOM},
101 {
"BBCOREPOINTER", Token::BBCOREPOINTER},
102 {
"EXTERNAL", Token::EXTERNAL},
103 {
"INCLUDE", Token::INCLUDE1},
104 {
"CONSTRUCTOR", Token::CONSTRUCTOR},
105 {
"DESTRUCTOR", Token::DESTRUCTOR},
106 {
"NET_RECEIVE", Token::NETRECEIVE},
107 {
"BEFORE", Token::BEFORE},
108 {
"AFTER", Token::AFTER},
109 {
"WATCH", Token::WATCH},
110 {
"FOR_NETCONS", Token::FOR_NETCONS},
111 {
"THREADSAFE", Token::THREADSAFE},
112 {
"PROTECT", Token::PROTECT},
113 {
"MUTEXLOCK", Token::NRNMUTEXLOCK},
114 {
"MUTEXUNLOCK", Token::NRNMUTEXUNLOCK}};
220 "state_discontinuity",
226 const static std::vector<std::string>
need_nt = {
"at_time"};
248 {
"t",
"dt",
"celsius",
"v",
"diam",
"area",
"pi",
"secondorder"};
289 return Token::METHOD;
291 throw std::runtime_error(
"token_type called for non-existent token " + name);
309 std::vector<std::string> result;
312 result.push_back(method.first);
314 result.insert(result.cend(),
Legacy macro definitions from mod2c/nocmodl implementation.
parser::NmodlParser::token_type TokenType
const static std::vector< std::string > extern_definitions
std::vector< std::string > get_external_functions()
Return functions that can be used in the NMODL.
NmodlParser::token_type TokenType
bool needs_neuron_thread_first_arg(const std::string &token)
Checks if token is one of the functions coming from NEURON/CoreNEURON and needs passing NrnThread* as...
encapsulates code generation backend implementations
TokenType keyword_type(const std::string &name)
Return token type for the keyword.
Map different tokens from lexer to token types.
MethodInfo(int64_t s, int v)
int variable_timestep
true if it is a variable timestep method
bool is_method(const std::string &name)
Check if given name is an integration method in NMODL.
bool is_keyword(const std::string &name)
Check if given name is a keyword in NMODL.
const static std::vector< std::string > need_nt
parser::CParser::token Token
Auto generated AST classes declaration.
const static std::map< std::string, TokenType > keywords
Keywords from NMODL language.
Information about integration method.
#define DERF
bit masks for block types where integration method are used
const static std::map< std::string, MethodInfo > methods
Integration methods available in the NMODL.
static const std::vector< std::string > NEURON_VARIABLES
Variables from NEURON that are directly used in NMODL.
TokenType token_type(const std::string &name)
Return token type for given token name.
std::vector< std::string > get_external_variables()
Return variables declared in NEURON that are available to NMODL.
int64_t subtype
block types where this method will work with