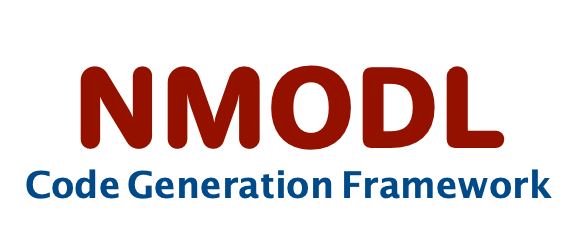 |
User Guide
|
Go to the documentation of this file.
17 #include "parser/nmodl/nmodl_parser.hpp"
std::vector< std::string > get_external_functions()
Return functions that can be used in the NMODL.
bool needs_neuron_thread_first_arg(const std::string &token)
Checks if token is one of the functions coming from NEURON/CoreNEURON and needs passing NrnThread* as...
encapsulates code generation backend implementations
bool is_method(const std::string &name)
Check if given name is an integration method in NMODL.
bool is_keyword(const std::string &name)
Check if given name is a keyword in NMODL.
TokenType token_type(const std::string &name)
Return token type for given token name.
std::vector< std::string > get_external_variables()
Return variables declared in NEURON that are available to NMODL.