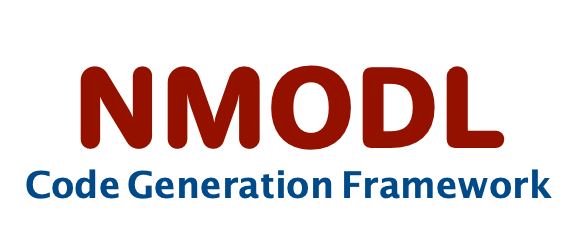 |
User Guide
|
Go to the documentation of this file.
26 #include <unordered_map>
98 explicit Unit(std::string name)
102 Unit(
const double factor,
const std::array<int, MAX_DIMS>& dimensions, std::string name)
110 void add_unit(
const std::string& name);
203 Prefix(std::string name,
const std::string& factor);
243 std::unordered_map<std::string, std::shared_ptr<Unit>>
table;
267 void insert(
const std::shared_ptr<Unit>& unit);
274 const std::shared_ptr<Unit>&
get_unit(
const std::string& unit_name)
const {
275 return table.at(unit_name);
void add_nominator_unit(const std::string &nom)
Add a unit to the vector of nominator strings of the Unit, so it can be processed later.
Class that stores all the data of a Unit.
UnitTable()=default
Default constructor for UnitTable.
const std::string & get_base_unit_name(int id) const noexcept
Get base unit name based on the ID number of the dimension.
const std::vector< std::string > & get_denominator_unit() const noexcept
Getter for the vector of denominators of the Unit.
double get_factor() const
Getter for the double factor of the Unit.
static constexpr int MAX_DIMS
Maximum number of dimensions of units (maximum number of base units)
std::unordered_map< std::string, std::shared_ptr< Unit > > table
Hash map that stores all the Units.
std::array< int, MAX_DIMS > unit_dimensions
Array of MAX_DIMS size that keeps the Unit's dimensions.
std::unordered_map< std::string, double > prefixes
Hash map that stores all the Prefixes.
double prefix_factor
Prefix's double factor.
void insert(const std::shared_ptr< Unit > &unit)
Insert a unit to the UnitTable table and calculate its dimensions and factor based on the previously ...
encapsulates code generation backend implementations
void add_nominator_dims(const std::array< int, MAX_DIMS > &dimensions)
Add the dimensions of a nominator of the unit to the dimensions of the Unit.
const std::vector< std::string > & get_nominator_unit() const noexcept
Getter for the vector of nominators of the Unit.
double get_factor() const noexcept
Getter for the factor of the Prefix.
void add_denominator_dims(const std::array< int, MAX_DIMS > &dimensions)
Subtract the dimensions of a nominator of the unit to the dimensions of the Unit.
double unit_factor
Double factor of the Unit.
void mul_factor(double double_factor)
Multiply Unit's factor with a double factor.
std::vector< std::string > nominator
Vector of nominators of the Unit.
Prefix()=delete
Default constructor for Prefix.
void add_unit(const std::string &name)
Add unit name to the Unit.
std::array< std::string, MAX_DIMS > base_units_names
Hash map that stores all the base units' names.
std::string unit_name
Name of the Unit.
void calc_denominator_dims(const std::shared_ptr< Unit > &unit, std::string denominator_name)
Calculate unit's dimensions based on its denominator unit named denominator_name which is stored in t...
std::string prefix_name
Prefix's name.
Unit(const double factor, const std::array< int, MAX_DIMS > &dimensions, std::string name)
Constructor that instantiates a Unit with its factor, dimensions and name.
void print_units_sorted(std::ostream &units_details) const
Print the details of the units that are stored in the UnitTable to the output stream units_details in...
Unit()=default
Default constructor of Unit.
void calc_nominator_dims(const std::shared_ptr< Unit > &unit, std::string nominator_name)
Calculate unit's dimensions based on its nominator unit named nominator_name which is stored in the U...
void print_base_units(std::ostream &base_units_details) const
Print the base units that are stored in the UnitTable to the output stream base_units_details.
Unit(std::string name)
Constructor for simply creating a Unit with a given name.
const std::shared_ptr< Unit > & get_unit(const std::string &unit_name) const
Get the unit_name of the UnitTable's table.
Class that stores all the units, prefixes and names of base units used.
void add_denominator_unit(const std::string &denom)
Add a unit to the vector of denominator strings of the Unit, so it can be processed later.
void insert_prefix(const std::shared_ptr< Prefix > &prfx)
Insert a prefix to the prefixes of the UnitTable.
std::vector< std::string > denominator
Vector of denominators of the Unit.
const std::string & get_name() const noexcept
Getter for the name of the Unit.
void add_fraction(const std::string &nominator, const std::string &denominator)
Parse a fraction given as string and store the result to the factor of the Unit.
static double parse_double(std::string double_string)
Parse a double number given as string.
void add_nominator_double(const std::string &double_string)
Takes as argument a double as string, parses it as double and stores it to the Unit factor.
void add_base_unit(const std::string &name)
If the Unit is a base unit the dimensions of the Unit should be calculated based on the name of the b...
const std::array< int, MAX_DIMS > & get_dimensions() const noexcept
Getter for the array of Unit's dimensions.
const std::string & get_name() const noexcept
Getter for the name of the Prefix.
Class that stores all the data of a prefix.