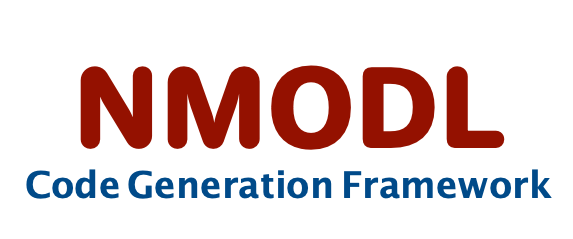 |
User Guide
|
Go to the documentation of this file.
45 class NonspecificCurVar;
46 class ElectrodeCurVar;
51 class BbcorePointerVar;
54 class IndependentBlock;
58 class ConstructorBlock;
59 class DestructorBlock;
61 class DerivativeBlock;
65 class FunctionTableBlock;
68 class NetReceiveBlock;
70 class BreakpointBlock;
87 class ReactionOperator;
88 class ParenExpression;
89 class BinaryExpression;
90 class DiffEqExpression;
91 class UnaryExpression;
101 class LocalListStatement;
106 class AssignedDefinition;
107 class ConductanceHint;
108 class ExpressionStatement;
109 class ProtectStatement;
111 class WhileStatement;
113 class ElseIfStatement;
115 class WatchStatement;
121 class ReactionStatement;
123 class ConstantStatement;
124 class TableStatement;
128 class ElectrodeCurrent;
139 class OntologyStatement;
142 class EigenNewtonSolverBlock;
143 class EigenLinearSolverBlock;
144 class WrappedExpression;
145 class DerivimplicitCallback;
146 class SolutionExpression;
std::vector< std::shared_ptr< ConstantBlock > > ConstantBlockVector
std::vector< std::shared_ptr< NonLinearBlock > > NonLinearBlockVector
std::vector< std::shared_ptr< UnaryExpression > > UnaryExpressionVector
@ RANDOM_VAR_LIST
type of ast::RandomVarList
std::vector< std::shared_ptr< ConstantStatement > > ConstantStatementVector
@ INDEXED_NAME
type of ast::IndexedName
@ WHILE_STATEMENT
type of ast::WhileStatement
@ DERIVATIVE_BLOCK
type of ast::DerivativeBlock
std::vector< std::shared_ptr< Conserve > > ConserveVector
std::vector< std::shared_ptr< Suffix > > SuffixVector
@ UNIT_STATE
type of ast::UnitState
@ REACT_VAR_NAME
type of ast::ReactVarName
@ RANDOM_VAR
type of ast::RandomVar
std::vector< std::shared_ptr< Compartment > > CompartmentVector
std::vector< std::shared_ptr< NumberRange > > NumberRangeVector
std::vector< std::shared_ptr< BinaryExpression > > BinaryExpressionVector
@ BINARY_EXPRESSION
type of ast::BinaryExpression
@ FUNCTION_BLOCK
type of ast::FunctionBlock
std::vector< std::shared_ptr< DiffEqExpression > > DiffEqExpressionVector
std::vector< std::shared_ptr< ConstantVar > > ConstantVarVector
std::vector< std::shared_ptr< NonLinEquation > > NonLinEquationVector
std::vector< std::shared_ptr< ExpressionStatement > > ExpressionStatementVector
@ MUTEX_UNLOCK
type of ast::MutexUnlock
std::vector< std::shared_ptr< Model > > ModelVector
@ DERIVIMPLICIT_CALLBACK
type of ast::DerivimplicitCallback
@ FOR_NETCON
type of ast::ForNetcon
std::vector< std::shared_ptr< ParamBlock > > ParamBlockVector
@ SOLUTION_EXPRESSION
type of ast::SolutionExpression
std::vector< std::shared_ptr< LinearBlock > > LinearBlockVector
@ KINETIC_BLOCK
type of ast::KineticBlock
@ COMPARTMENT
type of ast::Compartment
@ LAG_STATEMENT
type of ast::LagStatement
@ RANGE
type of ast::Range
@ INITIAL_BLOCK
type of ast::InitialBlock
std::vector< std::shared_ptr< PointerVar > > PointerVarVector
@ NONSPECIFIC_CUR_VAR
type of ast::NonspecificCurVar
@ MUTEX_LOCK
type of ast::MutexLock
std::vector< std::shared_ptr< EigenNewtonSolverBlock > > EigenNewtonSolverBlockVector
std::vector< std::shared_ptr< Statement > > StatementVector
@ MODEL
type of ast::Model
@ STATEMENT_BLOCK
type of ast::StatementBlock
@ FLOAT
type of ast::Float
std::vector< std::shared_ptr< ParamAssign > > ParamAssignVector
std::vector< std::shared_ptr< RangeVar > > RangeVarVector
std::vector< std::shared_ptr< TableStatement > > TableStatementVector
std::vector< std::shared_ptr< BlockComment > > BlockCommentVector
@ FUNCTION_TABLE_BLOCK
type of ast::FunctionTableBlock
@ EIGEN_NEWTON_SOLVER_BLOCK
type of ast::EigenNewtonSolverBlock
encapsulates code generation backend implementations
@ LIN_EQUATION
type of ast::LinEquation
std::vector< std::shared_ptr< Node > > NodeVector
std::vector< std::shared_ptr< UnitBlock > > UnitBlockVector
std::vector< std::shared_ptr< Block > > BlockVector
std::vector< std::shared_ptr< Pointer > > PointerVector
@ UPDATE_DT
type of ast::UpdateDt
@ CONSTRUCTOR_BLOCK
type of ast::ConstructorBlock
std::vector< std::shared_ptr< Double > > DoubleVector
std::vector< std::shared_ptr< External > > ExternalVector
std::vector< std::shared_ptr< BeforeBlock > > BeforeBlockVector
@ ELECTRODE_CURRENT
type of ast::ElectrodeCurrent
std::vector< std::shared_ptr< FactorDef > > FactorDefVector
std::vector< std::shared_ptr< RandomVar > > RandomVarVector
@ POINTER_VAR
type of ast::PointerVar
@ PRIME_NAME
type of ast::PrimeName
std::vector< std::shared_ptr< Boolean > > BooleanVector
@ ARGUMENT
type of ast::Argument
@ CONSERVE
type of ast::Conserve
@ PARAM_ASSIGN
type of ast::ParamAssign
std::vector< std::shared_ptr< ConstructorBlock > > ConstructorBlockVector
std::vector< std::shared_ptr< Number > > NumberVector
std::vector< std::shared_ptr< DestructorBlock > > DestructorBlockVector
std::vector< std::shared_ptr< FunctionTableBlock > > FunctionTableBlockVector
@ BLOCK_COMMENT
type of ast::BlockComment
@ DESTRUCTOR_BLOCK
type of ast::DestructorBlock
std::vector< std::shared_ptr< ReactVarName > > ReactVarNameVector
std::vector< std::shared_ptr< NeuronBlock > > NeuronBlockVector
std::vector< std::shared_ptr< Nonspecific > > NonspecificVector
std::vector< std::shared_ptr< WrappedExpression > > WrappedExpressionVector
@ BINARY_OPERATOR
type of ast::BinaryOperator
std::vector< std::shared_ptr< Verbatim > > VerbatimVector
std::vector< std::shared_ptr< LocalVar > > LocalVarVector
std::vector< std::shared_ptr< GlobalVar > > GlobalVarVector
AstNodeType
Enum type for every AST node type.
@ CONSTANT_VAR
type of ast::ConstantVar
std::vector< std::shared_ptr< ReactionStatement > > ReactionStatementVector
std::vector< std::shared_ptr< FunctionCall > > FunctionCallVector
@ PROCEDURE_BLOCK
type of ast::ProcedureBlock
@ BOOLEAN
type of ast::Boolean
@ READ_ION_VAR
type of ast::ReadIonVar
std::vector< std::shared_ptr< IndexedName > > IndexedNameVector
std::vector< std::shared_ptr< VarName > > VarNameVector
std::vector< std::shared_ptr< DiscreteBlock > > DiscreteBlockVector
@ DIFF_EQ_EXPRESSION
type of ast::DiffEqExpression
std::vector< std::shared_ptr< ReadIonVar > > ReadIonVarVector
std::vector< std::shared_ptr< ElseIfStatement > > ElseIfStatementVector
@ LON_DIFUSE
type of ast::LonDifuse
std::vector< std::shared_ptr< Name > > NameVector
@ DOUBLE
type of ast::Double
@ UNIT_BLOCK
type of ast::UnitBlock
@ IDENTIFIER
type of ast::Identifier
@ AFTER_BLOCK
type of ast::AfterBlock
std::vector< std::shared_ptr< OntologyStatement > > OntologyStatementVector
std::vector< std::shared_ptr< Argument > > ArgumentVector
std::vector< std::shared_ptr< UnitDef > > UnitDefVector
@ ELECTRODE_CUR_VAR
type of ast::ElectrodeCurVar
std::vector< std::shared_ptr< LagStatement > > LagStatementVector
std::vector< std::shared_ptr< LocalListStatement > > LocalListStatementVector
std::vector< std::shared_ptr< Program > > ProgramVector
std::vector< std::shared_ptr< AssignedBlock > > AssignedBlockVector
std::vector< std::shared_ptr< AfterBlock > > AfterBlockVector
std::vector< std::shared_ptr< BbcorePointerVar > > BbcorePointerVarVector
std::vector< std::shared_ptr< IfStatement > > IfStatementVector
std::vector< std::shared_ptr< String > > StringVector
@ CONDUCTANCE_HINT
type of ast::ConductanceHint
@ NONSPECIFIC
type of ast::Nonspecific
@ NUMBER
type of ast::Number
std::vector< std::shared_ptr< UnitState > > UnitStateVector
@ ONTOLOGY_STATEMENT
type of ast::OntologyStatement
@ ELSE_IF_STATEMENT
type of ast::ElseIfStatement
std::vector< std::shared_ptr< ElectrodeCurrent > > ElectrodeCurrentVector
std::vector< std::shared_ptr< ProcedureBlock > > ProcedureBlockVector
std::vector< std::shared_ptr< BreakpointBlock > > BreakpointBlockVector
@ SUFFIX
type of ast::Suffix
std::vector< std::shared_ptr< WhileStatement > > WhileStatementVector
std::vector< std::shared_ptr< MutexUnlock > > MutexUnlockVector
std::vector< std::shared_ptr< ConductanceHint > > ConductanceHintVector
std::vector< std::shared_ptr< Integer > > IntegerVector
@ NON_LINEAR_BLOCK
type of ast::NonLinearBlock
std::vector< std::shared_ptr< NrnStateBlock > > NrnStateBlockVector
@ STRING
type of ast::String
@ SOLVE_BLOCK
type of ast::SolveBlock
@ NRN_STATE_BLOCK
type of ast::NrnStateBlock
@ DEFINE
type of ast::Define
@ BA_BLOCK_TYPE
type of ast::BABlockType
std::vector< std::shared_ptr< RandomVarList > > RandomVarListVector
std::vector< std::shared_ptr< SolveBlock > > SolveBlockVector
std::vector< std::shared_ptr< StateBlock > > StateBlockVector
@ UNIT_DEF
type of ast::UnitDef
std::vector< std::shared_ptr< SolutionExpression > > SolutionExpressionVector
@ LINE_COMMENT
type of ast::LineComment
@ FUNCTION_CALL
type of ast::FunctionCall
std::vector< std::shared_ptr< Useion > > UseionVector
std::vector< std::shared_ptr< InitialBlock > > InitialBlockVector
@ UNARY_OPERATOR
type of ast::UnaryOperator
@ GLOBAL
type of ast::Global
std::vector< std::shared_ptr< BABlockType > > BABlockTypeVector
std::vector< std::shared_ptr< Include > > IncludeVector
std::vector< std::shared_ptr< ElectrodeCurVar > > ElectrodeCurVarVector
@ EXTERN_VAR
type of ast::ExternVar
@ LOCAL_VAR
type of ast::LocalVar
std::vector< std::shared_ptr< DerivimplicitCallback > > DerivimplicitCallbackVector
@ WATCH
type of ast::Watch
std::vector< std::shared_ptr< PrimeName > > PrimeNameVector
@ NEURON_BLOCK
type of ast::NeuronBlock
@ WATCH_STATEMENT
type of ast::WatchStatement
@ WRAPPED_EXPRESSION
type of ast::WrappedExpression
@ EXPRESSION_STATEMENT
type of ast::ExpressionStatement
std::vector< std::shared_ptr< LinEquation > > LinEquationVector
std::vector< std::shared_ptr< UpdateDt > > UpdateDtVector
@ EXPRESSION
type of ast::Expression
@ EIGEN_LINEAR_SOLVER_BLOCK
type of ast::EigenLinearSolverBlock
std::vector< std::shared_ptr< WatchStatement > > WatchStatementVector
@ PROTECT_STATEMENT
type of ast::ProtectStatement
std::vector< std::shared_ptr< DerivativeBlock > > DerivativeBlockVector
@ NUMBER_RANGE
type of ast::NumberRange
std::vector< std::shared_ptr< StatementBlock > > StatementBlockVector
@ WRITE_ION_VAR
type of ast::WriteIonVar
@ USEION
type of ast::Useion
@ VALENCE
type of ast::Valence
std::vector< std::shared_ptr< Expression > > ExpressionVector
@ REACTION_OPERATOR
type of ast::ReactionOperator
std::vector< std::shared_ptr< UnaryOperator > > UnaryOperatorVector
@ INTEGER
type of ast::Integer
std::vector< std::shared_ptr< AssignedDefinition > > AssignedDefinitionVector
std::vector< std::shared_ptr< NonspecificCurVar > > NonspecificCurVarVector
@ ASSIGNED_DEFINITION
type of ast::AssignedDefinition
std::vector< std::shared_ptr< BinaryOperator > > BinaryOperatorVector
std::vector< std::shared_ptr< KineticBlock > > KineticBlockVector
std::vector< std::shared_ptr< DoubleUnit > > DoubleUnitVector
@ POINTER
type of ast::Pointer
@ FACTOR_DEF
type of ast::FactorDef
@ PARAM_BLOCK
type of ast::ParamBlock
std::vector< std::shared_ptr< ParenExpression > > ParenExpressionVector
std::vector< std::shared_ptr< ForNetcon > > ForNetconVector
std::vector< std::shared_ptr< MutexLock > > MutexLockVector
@ INDEPENDENT_BLOCK
type of ast::IndependentBlock
@ CONSTANT_STATEMENT
type of ast::ConstantStatement
@ LOCAL_LIST_STATEMENT
type of ast::LocalListStatement
std::vector< std::shared_ptr< Watch > > WatchVector
std::vector< std::shared_ptr< BABlock > > BABlockVector
std::vector< std::shared_ptr< FromStatement > > FromStatementVector
@ STATEMENT
type of ast::Statement
@ VERBATIM
type of ast::Verbatim
@ INCLUDE
type of ast::Include
@ UNARY_EXPRESSION
type of ast::UnaryExpression
@ STATE_BLOCK
type of ast::StateBlock
@ DISCRETE_BLOCK
type of ast::DiscreteBlock
std::vector< std::shared_ptr< LonDifuse > > LonDifuseVector
std::vector< std::shared_ptr< NetReceiveBlock > > NetReceiveBlockVector
std::vector< std::shared_ptr< Identifier > > IdentifierVector
@ GLOBAL_VAR
type of ast::GlobalVar
std::vector< std::shared_ptr< BbcorePointer > > BbcorePointerVector
@ THREAD_SAFE
type of ast::ThreadSafe
std::vector< std::shared_ptr< Limits > > LimitsVector
@ BLOCK
type of ast::Block
@ PAREN_EXPRESSION
type of ast::ParenExpression
@ BEFORE_BLOCK
type of ast::BeforeBlock
std::vector< std::shared_ptr< ElseStatement > > ElseStatementVector
@ BBCORE_POINTER
type of ast::BbcorePointer
std::vector< std::shared_ptr< Global > > GlobalVector
std::vector< std::shared_ptr< ProtectStatement > > ProtectStatementVector
@ DOUBLE_UNIT
type of ast::DoubleUnit
std::vector< std::shared_ptr< FunctionBlock > > FunctionBlockVector
std::vector< std::shared_ptr< WriteIonVar > > WriteIonVarVector
@ TABLE_STATEMENT
type of ast::TableStatement
@ NON_LIN_EQUATION
type of ast::NonLinEquation
std::vector< std::shared_ptr< EigenLinearSolverBlock > > EigenLinearSolverBlockVector
std::vector< std::shared_ptr< ThreadSafe > > ThreadSafeVector
@ ASSIGNED_BLOCK
type of ast::AssignedBlock
@ BBCORE_POINTER_VAR
type of ast::BbcorePointerVar
std::vector< std::shared_ptr< Range > > RangeVector
@ BREAKPOINT_BLOCK
type of ast::BreakpointBlock
std::vector< std::shared_ptr< LineComment > > LineCommentVector
std::vector< std::shared_ptr< IndependentBlock > > IndependentBlockVector
std::vector< std::shared_ptr< ExternVar > > ExternVarVector
std::vector< std::shared_ptr< Float > > FloatVector
@ FROM_STATEMENT
type of ast::FromStatement
@ BA_BLOCK
type of ast::BABlock
@ NET_RECEIVE_BLOCK
type of ast::NetReceiveBlock
std::vector< std::shared_ptr< Valence > > ValenceVector
@ PROGRAM
type of ast::Program
@ RANGE_VAR
type of ast::RangeVar
std::vector< std::shared_ptr< Define > > DefineVector
@ LIMITS
type of ast::Limits
@ REACTION_STATEMENT
type of ast::ReactionStatement
@ CONSTANT_BLOCK
type of ast::ConstantBlock
@ EXTERNAL
type of ast::External
std::vector< std::shared_ptr< Unit > > UnitVector
@ ELSE_STATEMENT
type of ast::ElseStatement
@ VAR_NAME
type of ast::VarName
std::vector< std::shared_ptr< ReactionOperator > > ReactionOperatorVector
@ IF_STATEMENT
type of ast::IfStatement
@ LINEAR_BLOCK
type of ast::LinearBlock