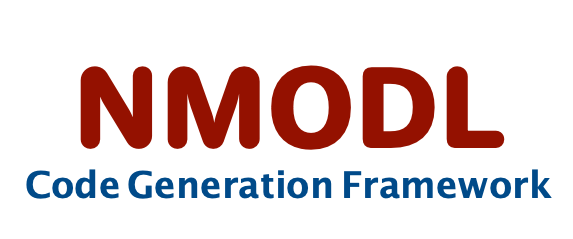 |
User Guide
|
Go to the documentation of this file.
11 #include <unordered_map>
183 {
"_ppvar",
"indexes"},
184 {
"_thread",
"thread"},
186 {
"_cntml_padded",
"pnodecount"},
187 {
"_cntml",
"nodecount"},
188 {
"_tqitem",
"tqitem"}};
193 {
"random_setseq",
"nrnran123_setseq"},
194 {
"random_setids",
"nrnran123_setids"},
195 {
"random_uniform",
"nrnran123_uniform"},
196 {
"random_negexp",
"nrnran123_negexp"},
197 {
"random_normal",
"nrnran123_normal"},
198 {
"random_ipick",
"nrnran123_ipick"},
199 {
"random_dpick",
"nrnran123_dblpick"}};
static constexpr char RANDOM_SEMANTIC[]
semantic type for RANDOM variable
static constexpr char NET_SEND_SEMANTIC[]
semantic type for net send call
static constexpr char CELSIUS_VARIABLE[]
global temperature variable
static constexpr char DEFAULT_LOCAL_VAR_TYPE[]
default local variable type
static constexpr char CNEXP_METHOD[]
cnexp method in nmodl
constexpr char NRN_PRIVATE_DESTRUCTOR_METHOD[]
nrn_private_destructor method in generated code
static constexpr char POINT_PROCESS_VARIABLE[]
inbuilt neuron variable for point process
static constexpr char NRN_JACOB_METHOD[]
nrn_jacob method in generated code
static constexpr char NTHREAD_D_SHADOW[]
shadow d variable in neuron thread structure
static constexpr char VOLTAGE_UNUSED_VARIABLE[]
range variable for voltage when unused (for vectorized model)
static constexpr char NRN_WATCH_CHECK_METHOD[]
nrn_watch_check method in generated c++ file
static constexpr char POINT_PROCESS[]
point process keyword in nmodl
encapsulates code generation backend implementations
static constexpr char NRN_CONSTRUCTOR_METHOD[]
nrn_constructor method in generated code
static constexpr char NTHREAD_DT_VARIABLE[]
dt variable in neuron thread structure
static constexpr char NODE_AREA_VARIABLE[]
inbuilt neuron variable for area of the compartment
static constexpr char NRN_ALLOC_METHOD[]
nrn_alloc method in generated code
static constexpr char SPARSE_METHOD[]
sparse method in nmodl
static constexpr char ARTIFICIAL_CELL[]
artificial cell keyword in nmodl
static constexpr char CONDUCTANCE_VARIABLE[]
range variable for conductance
static constexpr char DEFAULT_FLOAT_TYPE[]
default float variable type
static constexpr char NTHREAD_RHS_SHADOW[]
shadow rhs variable in neuron thread structure
static constexpr char USE_TABLE_VARIABLE[]
global variable to indicate if table is used
static constexpr char POINT_PROCESS_SEMANTIC[]
semantic type for point process variable
static const std::unordered_map< std::string, std::string > VERBATIM_VARIABLES_MAPPING
commonly used variables in verbatim block and how they should be mapped to new code generation backen...
static constexpr char NMODL_VERSION[]
nmodl language version
static constexpr char NRN_INIT_METHOD[]
nrn_init method in generated code
static constexpr char THREAD_ARGS[]
verbatim name of the variable for nrn thread arguments
static constexpr char POINTER_SEMANTIC[]
semantic type for pointer variable
static constexpr char AREA_SEMANTIC[]
semantic type for area variable
static constexpr char NRN_CUR_METHOD[]
nrn_cur method in generated code
static std::unordered_map< std::string, std::string > RANDOM_FUNCTIONS_MAPPING
static constexpr char TQITEM_VARIABLE[]
inbuilt neuron variable for tqitem process
static constexpr char DEFAULT_INTEGER_TYPE[]
default integer variable type
static constexpr char INST_GLOBAL_MEMBER[]
instance struct member pointing to the global variable structure
static constexpr char CORE_POINTER_SEMANTIC[]
semantic type for core pointer variable
static constexpr char WATCH_SEMANTIC[]
semantic type for watch statement
static constexpr char DERIVIMPLICIT_METHOD[]
derivimplicit method in nmodl
static constexpr char NET_MOVE_METHOD[]
net_move function call in nmodl
static constexpr char EULER_METHOD[]
euler method in nmodl
static constexpr char NET_EVENT_METHOD[]
net_event function call in nmodl
static constexpr char NRN_STATE_METHOD[]
nrn_state method in generated code
static constexpr char AREA_VARIABLE[]
similar to node_area but user can explicitly declare it as area
static constexpr char AFTER_CVODE_METHOD[]
cvode method in nmodl
static constexpr char T_SAVE_VARIABLE[]
variable t indicating last execution time of net receive block
static constexpr char FOR_NETCON_SEMANTIC[]
semantic type for for_netcon statement
static constexpr char NTHREAD_T_VARIABLE[]
t variable in neuron thread structure
static constexpr char DIAM_VARIABLE[]
inbuilt neuron variable for diameter of the compartment
static constexpr char NRN_DESTRUCTOR_METHOD[]
nrn_destructor method in generated code
static constexpr char CONDUCTANCE_UNUSED_VARIABLE[]
range variable when conductance is not used (for vectorized model)
static constexpr char NRN_POINTERINDEX[]
hoc_nrnpointerindex name
static constexpr char THREAD_ARGS_PROTO[]
verbatim name of the variable for nrn thread arguments in prototype
constexpr char NRN_PRIVATE_CONSTRUCTOR_METHOD[]
nrn_private_constructor method in generated code
static constexpr char NET_SEND_METHOD[]
net_send function call in nmodl
static constexpr char ION_VARNAME_PREFIX[]
prefix for ion variable