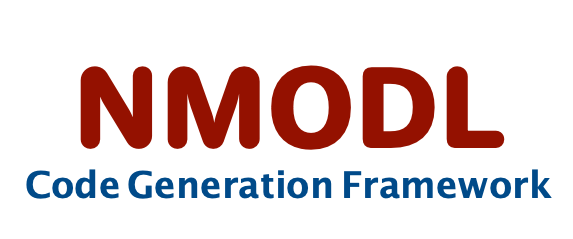 |
User Guide
|
LocalizeVisitor()=default
LocalizeVisitor(bool ignore_verbatim)
Base class for all AST node.
Concrete constant visitor for all AST classes.
std::vector< std::string > variables_to_optimize() const
encapsulates code generation backend implementations
Visitor to transform global variable usage to local
bool ignore_verbatim
ignore verbatim blocks while localizing
bool is_solve_procedure(const ast::Node &node) const
void visit_program(const ast::Program &node) override
visit node of type ast::Program
Represent symbol table for a NMODL block.
bool node_for_def_use_analysis(const ast::Node &node) const
Represents top level AST node for whole NMODL input.
symtab::SymbolTable * program_symtab
Forward declarations of symbols in namespace nmodl::symtab.
Concrete visitor for all AST classes.