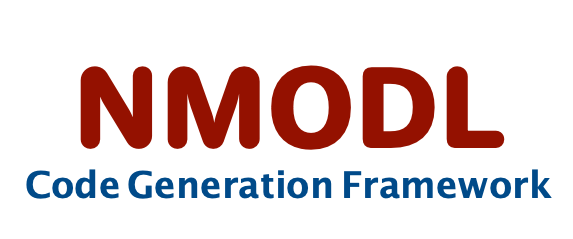 |
User Guide
|
Go to the documentation of this file.
20 #include "parser/nmodl/location.hh"
21 #include "parser/nmodl/nmodl_parser.hpp"
27 using Token = parser::NmodlParser::token;
parser::NmodlParser::token_type TokenType
SymbolType string_symbol(const std::string &text, PositionType &pos)
Create symbol for ast::String AST class.
parser::NmodlParser::token Token
SymbolType double_symbol(const std::string &value, PositionType &pos)
Create a symbol for ast::Double AST class.
encapsulates code generation backend implementations
parser::NmodlParser::symbol_type SymbolType
SymbolType integer_symbol(int value, PositionType &pos, const char *text)
Create a symbol for ast::Integer AST.
parser::location PositionType
SymbolType token_symbol(const std::string &key, PositionType &pos, TokenType type)
Create symbol for AST class.
SymbolType prime_symbol(std::string text, PositionType &pos)
Create symbol for ast::Prime AST class.
void symbol_type(const std::string &name, T &value)
SymbolType name_symbol(const std::string &text, PositionType &pos, TokenType type)
Create symbol for ast::Name AST class.
TokenType token_type(const std::string &name)
Return token type for given token name.