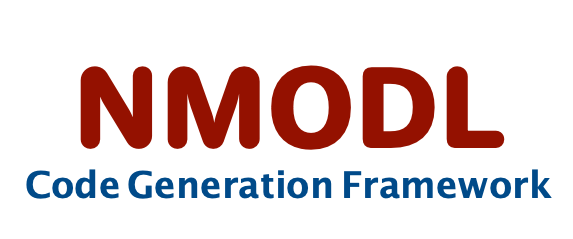 |
User Guide
|
Go to the documentation of this file.
57 const std::shared_ptr<ast::SolveBlock>& solve_block,
58 const std::vector<std::shared_ptr<ast::Ast>>& deriv_blocks);
const double STEADYSTATE_DERIVIMPLICIT_DT
encapsulates code generation backend implementations
std::shared_ptr< ast::DerivativeBlock > create_steadystate_block(const std::shared_ptr< ast::SolveBlock > &solve_block, const std::vector< std::shared_ptr< ast::Ast >> &deriv_blocks)
create new steady state derivative block for given solve block
const double STEADYSTATE_SPARSE_DT
Visitor for STEADYSTATE solve statements
SteadystateVisitor()=default
Concrete visitor for all AST classes.
void visit_program(ast::Program &node) override
visit node of type ast::Program
Represents top level AST node for whole NMODL input.
Concrete visitor for all AST classes.