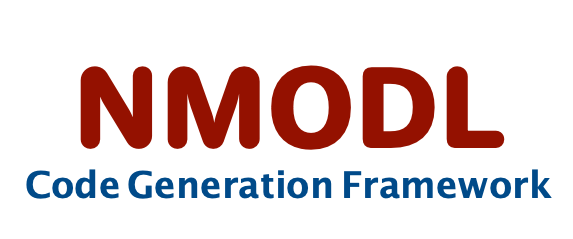 |
User Guide
|
const std::string UNIT_FUZZ
Declaration of fuzz constant unit, which is the equivilant of 1 in mod files UNITS definitions.
void visit_program(ast::Program &node) override
Override visit_program function to parse the nrnunits.lib unit file before starting visiting the AST ...
encapsulates code generation backend implementations
parser::UnitDriver units_driver
Units Driver needed to parse the units file and the string produces by mod files' units.
Utility functions for visitors implementation.
void visit_factor_def(ast::FactorDef &node) override
Function to visit all the ast::FactorDef nodes and parse the units defined as ast::FactorDef in the U...
UnitsVisitor()=default
Default UnitsVisitor constructor.
Concrete visitor for all AST classes.
const parser::UnitDriver & get_unit_driver() const noexcept
Get the parser::UnitDriver to be able to use it outside the visitor::UnitsVisitor scope keeping the s...
std::string units_dir
Directory of units lib file that defines all the basic units.
void visit_unit_def(ast::UnitDef &node) override
Function to visit all the ast::UnitDef nodes and parse the units defined as ast::UnitDef in the UNITS...
Visitor for Units blocks of AST.
Represents top level AST node for whole NMODL input.
Class that binds all pieces together for parsing C units.
UnitsVisitor(std::string t_units_dir)
UnitsVisitor constructor that takes as argument the units file to parse the units from.
Concrete visitor for all AST classes.