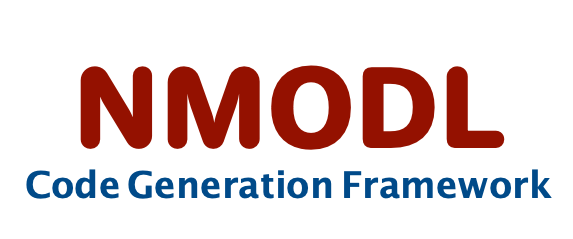 |
User Guide
|
Go to the documentation of this file.
26 if (current_symtab !=
nullptr) {
53 bool rename_plausible =
false;
57 rename_plausible =
true;
61 rename_plausible =
true;
65 rename_plausible =
true;
67 if (rename_plausible) {
69 if (symbol !=
nullptr) {
72 logger->warn(
"could not find {} definition in nmodl", name);
83 const auto& text = statement->eval();
87 const auto& tokens =
driver.all_tokens();
89 std::ostringstream oss;
90 for (
const auto& token: tokens) {
93 statement->set(oss.str());
const std::string ION_PREFIX
prefix used for range variables
Represents a C code block.
void visit_verbatim(ast::Verbatim &node) override
Parse verbatim blocks and rename variables used.
symtab::SymbolTable * symtab
non-null symbol table in the scope hierarchy
std::string rename_variable(const std::string &name)
Rename variable used in verbatim block if defined in NMODL scope.
Auto generated AST classes declaration.
encapsulates code generation backend implementations
std::shared_ptr< String > get_statement() const noexcept
Getter for member variable Verbatim::statement.
Class that binds all pieces together for parsing C verbatim blocks.
const StatementVector & get_statements() const noexcept
Getter for member variable StatementBlock::statements.
Auto generated AST classes declaration.
Auto generated AST classes declaration.
nmodl::parser::UnitDriver driver
Rename variable in verbatim block.
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
Represents block encapsulating list of statements.
void visit_statement_block(ast::StatementBlock &node) override
visit node of type ast::StatementBlock
std::stack< symtab::SymbolTable * > symtab_stack
symbol tables in nested blocks
Implement logger based on spdlog library.
const std::string RANGE_PREFIX
prefix used for range variables
std::shared_ptr< Symbol > lookup_in_scope(const std::string &name) const
check if symbol with given name exist in the current table (including all parents)
const std::string LOCAL_PREFIX
prefix used for local variable