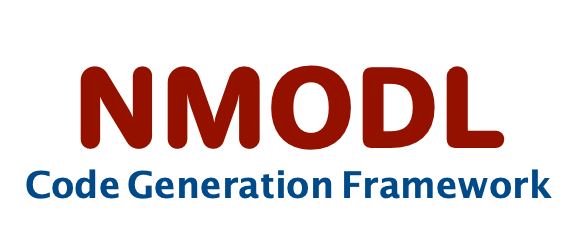 |
User Guide
|
Go to the documentation of this file.
34 std::string
a =
"0.0";
35 std::string
b =
"0.0";
39 Term(
const std::string&
expr,
const std::string& state);
50 return deriv !=
"0.0";
bool deriv_nonzero() const
helper routines used in parser
const std::string & state_variable() const
return the state variable
std::string get_euler_derivate() const
return only the derivate for euler method
Term(std::string expr, std::string deriv, std::string a, std::string b)
Term solution
"final" solution of the equation
std::string expr
expression being solved
std::string get_cnexp_solution() const
return solution for cnexp method
encapsulates code generation backend implementations
bool deriv_invalid
if derivative of the equation is invalid
Helper class used by driver and parser while solving diff equation.
std::string deriv
derivative of the expression
std::string get_cvode_linear_diffeq() const
for non-cnexp methods : return the solution based on if equation is linear or not
std::string rhs
rhs of equation
std::string get_expr_for_nonlinear() const
return expression with Dstate added
std::string method
name of the solve method
std::string cvode_eqnrhs() const
Represent a term in differential equation and it's "current" solution.
std::string get_solution(bool &cnexp_possible)
return solution of the differential equation
std::string cvode_deriv() const
bool eqn_invalid
if equation itself is invalid
std::string get_cvode_nonlinear_diffeq() const
Return solution for non-cnexp method and when equation is non-linear.
void print() const
print the context (for debugging)
std::string state
name of the state variable
DiffEqContext(std::string state, int, std::string rhs, std::string method)
std::string get_non_cnexp_solution() const
return solution for non-cnexp method