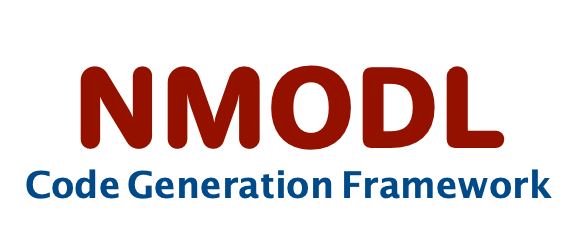 |
User Guide
|
Go to the documentation of this file.
18 Term::Term(
const std::string& expr,
const std::string& state)
30 std::cout <<
"Term [expr, deriv, a, b] : " <<
expr <<
", " <<
deriv <<
", " <<
a <<
", " <<
b
36 std::cout <<
"-----------------DiffEq Context----------------" << std::endl;
37 std::cout <<
"deriv_invalid = " <<
deriv_invalid << std::endl;
38 std::cout <<
"eqn_invalid = " <<
eqn_invalid << std::endl;
41 std::cout <<
"a = " <<
solution.
a << std::endl;
42 std::cout <<
"b = " <<
solution.
b << std::endl;
43 std::cout <<
"-----------------------------------------------" << std::endl;
71 std::string expression;
74 std::istringstream in(
rhs);
81 if (
token_type == DiffeqParser::by_type(DiffeqParser::token::END).type_get()) {
85 auto value = sym.value.as<std::string>();
87 expression +=
"(" + value +
"+0.001)";
110 std::string sol =
"D" +
state +
" = " +
"D" +
state +
"/(1.0-dt*(";
111 sol +=
"((" + expr +
")-(" +
solution.
expr +
"))/0.001))";
129 std::string suffix =
"/(0.0)";
130 b = b.substr(0, b.size() - suffix.size());
131 result =
state +
" = " +
state +
"-dt*(" + b +
")";
133 result =
state +
" = " +
state +
"+(1.0-exp(dt*(" + a +
")))*(" + b +
"-" +
state +
")";
157 throw std::runtime_error(
"Error in differential equation solver with non-cnexp");
173 cnexp_possible =
false;
176 cnexp_possible =
true;
179 cnexp_possible =
false;
std::string get_euler_derivate() const
return only the derivate for euler method
Term solution
"final" solution of the equation
std::string expr
expression being solved
Represent Lexer/Scanner class for differential equation parsing.
std::string get_cnexp_solution() const
return solution for cnexp method
encapsulates code generation backend implementations
Implement string manipulation functions.
bool deriv_invalid
if derivative of the equation is invalid
std::string deriv
derivative of the expression
std::string get_cvode_linear_diffeq() const
for non-cnexp methods : return the solution based on if equation is linear or not
std::string rhs
rhs of equation
std::string get_expr_for_nonlinear() const
return expression with Dstate added
std::string method
name of the solve method
std::string cvode_eqnrhs() const
virtual DiffeqParser::symbol_type next_token()
Function for lexer to scan token (replacement for yylex())
std::string get_solution(bool &cnexp_possible)
return solution of the differential equation
std::string cvode_deriv() const
bool eqn_invalid
if equation itself is invalid
std::string get_cvode_nonlinear_diffeq() const
Return solution for non-cnexp method and when equation is non-linear.
void print() const
print the context (for debugging)
std::string state
name of the state variable
TokenType token_type(const std::string &name)
Return token type for given token name.
std::string get_non_cnexp_solution() const
return solution for non-cnexp method