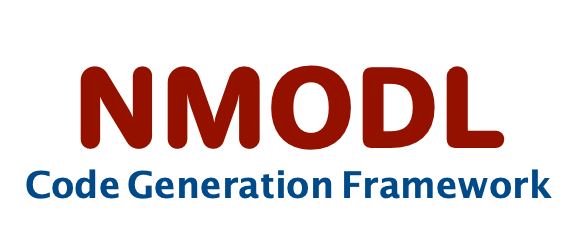 |
User Guide
|
Go to the documentation of this file.
11 #include <nlohmann/json.hpp>
18 if (filename.empty()) {
19 throw std::runtime_error(
"Empty filename for JSONPrinter");
22 ofs.open(filename.c_str());
25 auto msg =
"Error while opening file '" + filename +
"' for JSONPrinter";
26 throw std::runtime_error(msg);
36 throw std::logic_error{
"Block not initialized (push_block missing?)"};
40 j[key] = std::move(value);
41 block->front().push_back(std::move(j));
46 if (
block ==
nullptr) {
47 logger->warn(
"JSONPrinter : can't add property without block");
50 (*block)[name] = value;
65 j[value] = json::array();
67 block = std::shared_ptr<json>(
new json(j));
75 block->front().push_back(*current);
std::shared_ptr< json > block
single (current) nmodl block / statement
JSONPrinter()
By default dump output to std::cout.
encapsulates code generation backend implementations
std::shared_ptr< std::ostream > result
common output stream for file, cout or stringstream
const std::string child_key
json key for children
Helper class for printing AST in JSON form.
void add_node(std::string value, const std::string &key="name")
Add node to json (typically basic type)
bool expand
true if we need to expand keys i.e.
void push_block(const std::string &value, const std::string &key="name")
Add new json object (typically start of new block) name here is type of new block encountered.
void pop_block()
We finished processing a block, add processed block to it's parent block.
bool compact
true if need to print json in compact format
void flush()
Dump json object to stream (typically at the end) nspaces is number of spaces used for indentation.
void add_block_property(std::string const &name, const std::string &value)
Add property to the block which is added last.
std::stack< std::shared_ptr< json > > stack
stack that holds all parent blocks / statements
Implement logger based on spdlog library.