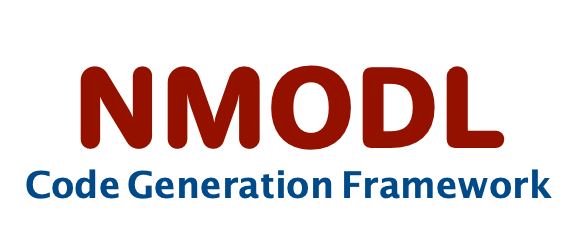 |
User Guide
|
Go to the documentation of this file.
15 #include <nlohmann/json_fwd.hpp>
49 std::streambuf*
sbuf =
nullptr;
52 std::shared_ptr<std::ostream>
result;
58 std::stack<std::shared_ptr<json>>
stack;
75 :
result(new std::ostream(std::cout.rdbuf())) {}
79 :
result(new std::ostream(os.rdbuf())) {}
85 void push_block(
const std::string& value,
const std::string& key =
"name");
86 void add_node(std::string value,
const std::string& key =
"name");
std::shared_ptr< json > block
single (current) nmodl block / statement
JSONPrinter()
By default dump output to std::cout.
encapsulates code generation backend implementations
std::shared_ptr< std::ostream > result
common output stream for file, cout or stringstream
const std::string child_key
json key for children
void add_node(std::string value, const std::string &key="name")
Add node to json (typically basic type)
Helper class for printing AST in JSON form.
bool expand
true if we need to expand keys i.e.
void compact_json(bool flag)
print json in compact mode
void push_block(const std::string &value, const std::string &key="name")
Add new json object (typically start of new block) name here is type of new block encountered.
void pop_block()
We finished processing a block, add processed block to it's parent block.
JSONPrinter(std::ostream &os)
bool compact
true if need to print json in compact format
void flush()
Dump json object to stream (typically at the end) nspaces is number of spaces used for indentation.
void add_block_property(std::string const &name, const std::string &value)
Add property to the block which is added last.
std::stack< std::shared_ptr< json > > stack
stack that holds all parent blocks / statements
void expand_keys(bool flag)