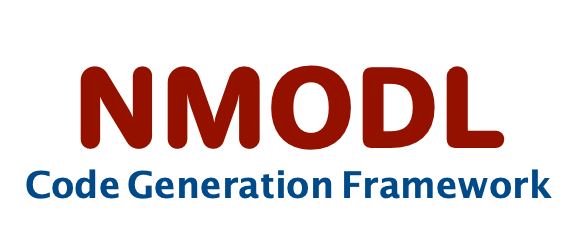 |
User Guide
|
Go to the documentation of this file.
102 void print(std::stringstream& stream)
const;
104 static std::vector<std::string>
keys();
106 std::vector<std::string>
values()
const;
int n_ext_func_call
could be external math funcs
std::vector< std::string > values() const
encapsulates code generation backend implementations
static std::vector< std::string > keys()
int n_global_read
expensive : typically access to dynamically allocated memory
int n_unique_constant_write
void print(std::stringstream &stream) const
int n_local_read
cheap : typically local variables in mod file means registers
int n_constant_read
could be optimized : access to variables that could be read-only in this case write counts are typica...
friend PerfStat operator+(const PerfStat &first, const PerfStat &second)
int n_int_func_call
mod functions (before/after inlining)
Helper class to collect performance statistics.
int n_unique_global_write
std::string title
name for pretty-printing
int n_exp
expensive functions : commonly used functions in mod files
int n_unique_constant_read
int n_add
basic ops (<= 1 cycle)