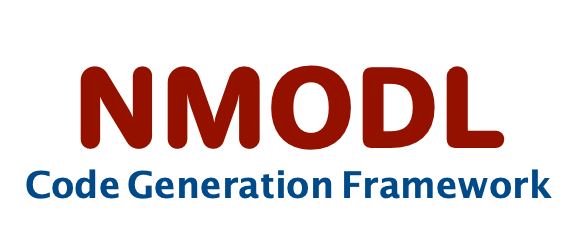 |
User Guide
|
Go to the documentation of this file.
77 return {
"+",
"-",
"x",
"/",
"exp",
"log",
"GM-R(T)",
78 "GM-R(U)",
"GM-W(T)",
"GM-W(U)",
"CM-R(T)",
"CM-R(U)",
"CM-W(T)",
"CM-W(U)",
79 "LM-R(T)",
"LM-W(T)",
"calls(ext)",
"calls(int)",
"compare",
"unary",
"conditional"};
83 std::vector<std::string> row;
int n_ext_func_call
could be external math funcs
std::string title
title of the table
std::vector< std::string > values() const
std::vector< TableRowType > rows
data
encapsulates code generation backend implementations
PerfStat operator+(const PerfStat &first, const PerfStat &second)
static std::vector< std::string > keys()
int n_global_read
expensive : typically access to dynamically allocated memory
Class to construct and pretty-print tabular data.
int n_unique_constant_write
Implement generic table data structure.
void print(std::stringstream &stream) const
int n_local_read
cheap : typically local variables in mod file means registers
std::string to_string(const T &obj)
int n_constant_read
could be optimized : access to variables that could be read-only in this case write counts are typica...
TableRowType headers
top header/keys
void print(int indent=0) const
int n_int_func_call
mod functions (before/after inlining)
Helper class to collect performance statistics.
int n_unique_global_write
Implement class for performance statistics.
std::string title
name for pretty-printing
int n_exp
expensive functions : commonly used functions in mod files
int n_unique_constant_read
int n_add
basic ops (<= 1 cycle)