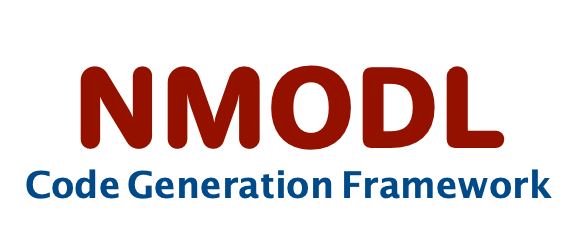 |
User Guide
|
Go to the documentation of this file.
232 template <
typename T>
235 return static_cast<T
>(~static_cast<utype>(arg));
238 template <
typename T>
241 return static_cast<T
>(
static_cast<utype
>(lhs) |
static_cast<utype
>(rhs));
244 template <
typename T>
247 return static_cast<T
>(
static_cast<utype
>(lhs) &
static_cast<utype
>(rhs));
250 template <
typename T>
256 template <
typename T>
264 return static_cast<bool>(obj & property);
269 return static_cast<bool>(obj & state);
281 template <
typename T>
290 text +=
" " + element;
bool has_status(const Status &obj, Status state)
check if any status is set
VariableType
usage of mod file as array or scalar
@ from_state
derived from state
@ write
variable is written only
@ write_ion_var
Write Ion.
DeclarationType
kind of symbol
@ use_range_ptr_var
FUNCTION or PROCEDURE needs setdata check.
@ extern_method
neuron solver methods and math functions
@ globalized
converted to global
@ thread_safe
variable marked as thread safe
@ table_assigned_var
variable is used in table as assigned
@ linear_block
Linear Block.
@ read
variable is ready only
encapsulates code generation backend implementations
@ constant_var
constant variable
@ to_solve
need to solve : used in solve statement
@ function_table_block
FunctionTable Block.
T operator&(T lhs, T rhs)
@ local_var
Local Variable.
Status
state during various compiler passes
Implement string manipulation functions.
@ codegen_var
Codegen specific variable.
@ scalar
scalar / single value
@ range_var
Range Variable.
std::ostream & operator<<(std::ostream &os, const NmodlType &obj)
@ kinetic_block
Kinetic Block.
@ function_block
Function Type.
@ table_statement_var
variable is used in table statement
@ procedure_block
Procedure Type.
@ define
Define variable / macro.
@ factor_def
factor in unit block
std::string to_string(const T &obj)
T & operator&=(T &lhs, T rhs)
Scope
scope within a mod file
@ nonspecific_cur_var
Non Specific Current.
Access
variable usage within a mod file
@ assigned_definition
Assigned Definition.
@ param_assign
Parameter Variable.
NmodlType
NMODL variable properties.
@ pointer_var
Pointer Type.
T & operator|=(T &lhs, T rhs)
@ longitudinal_diffusion_block
Internal LONGITUDINAL_DIFFUSION block.
@ bbcore_pointer_var
Bbcorepointer Type.
@ state_var
state variable
@ derivative_block
Derivative Block.
@ external
extern variable
@ non_linear_block
NonLinear Block.
@ global_var
Global Variable.
std::vector< std::string > to_string_vector(const NmodlType &obj)
helper function to convert nmodl properties to string
@ random_var
Randomvar Type.
T operator|(T lhs, T rhs)
@ localized
converted to local
@ discrete_block
Discrete Block.
@ extern_neuron_variable
neuron variable accessible in mod file
@ electrode_cur_var
Electrode Current.
bool has_property(const NmodlType &obj, NmodlType property)
check if any property is set