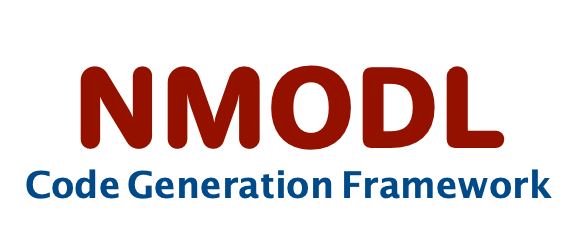 |
User Guide
|
Go to the documentation of this file.
9 #include <unordered_map>
24 std::vector<std::string> properties;
29 properties.emplace_back(
"local");
33 properties.emplace_back(
"global");
37 properties.emplace_back(
"range");
41 properties.emplace_back(
"parameter");
45 properties.emplace_back(
"pointer");
49 properties.emplace_back(
"bbcore_pointer");
53 properties.emplace_back(
"extern");
57 properties.emplace_back(
"prime_name");
61 properties.emplace_back(
"assigned_definition");
65 properties.emplace_back(
"unit_def");
69 properties.emplace_back(
"read_ion");
73 properties.emplace_back(
"write_ion");
77 properties.emplace_back(
"nonspecific_cur_var");
81 properties.emplace_back(
"electrode_cur");
85 properties.emplace_back(
"argument");
89 properties.emplace_back(
"function_block");
93 properties.emplace_back(
"procedure_block");
97 properties.emplace_back(
"derivative_block");
101 properties.emplace_back(
"linear_block");
105 properties.emplace_back(
"non_linear_block");
109 properties.emplace_back(
"table_statement_var");
113 properties.emplace_back(
"table_assigned_var");
117 properties.emplace_back(
"constant");
121 properties.emplace_back(
"kinetic_block");
125 properties.emplace_back(
"function_table_block");
129 properties.emplace_back(
"factor_def");
133 properties.emplace_back(
"extern_neuron_var");
137 properties.emplace_back(
"extern_method");
141 properties.emplace_back(
"state_var");
145 properties.emplace_back(
"to_solve");
149 properties.emplace_back(
"ion");
153 properties.emplace_back(
"discrete_block");
157 properties.emplace_back(
"define");
161 properties.emplace_back(
"codegen_var");
165 properties.emplace_back(
"use_range_ptr_var");
169 properties.emplace_back(
"random_var");
176 std::vector<std::string> status;
181 status.emplace_back(
"localized");
185 status.emplace_back(
"globalized");
189 status.emplace_back(
"inlined");
193 status.emplace_back(
"renamed");
197 status.emplace_back(
"created");
201 status.emplace_back(
"from_state");
205 status.emplace_back(
"thread_safe");
bool has_status(const Status &obj, Status state)
check if any status is set
@ from_state
derived from state
@ write_ion_var
Write Ion.
@ use_range_ptr_var
FUNCTION or PROCEDURE needs setdata check.
@ extern_method
neuron solver methods and math functions
@ globalized
converted to global
@ thread_safe
variable marked as thread safe
@ table_assigned_var
variable is used in table as assigned
@ linear_block
Linear Block.
encapsulates code generation backend implementations
@ constant_var
constant variable
@ to_solve
need to solve : used in solve statement
@ function_table_block
FunctionTable Block.
@ local_var
Local Variable.
Implement various classes to represent various Symbol properties.
Status
state during various compiler passes
Implement string manipulation functions.
@ codegen_var
Codegen specific variable.
@ range_var
Range Variable.
std::ostream & operator<<(std::ostream &os, const NmodlType &obj)
@ kinetic_block
Kinetic Block.
@ function_block
Function Type.
@ table_statement_var
variable is used in table statement
@ procedure_block
Procedure Type.
@ define
Define variable / macro.
@ factor_def
factor in unit block
std::string to_string(const T &obj)
@ nonspecific_cur_var
Non Specific Current.
@ assigned_definition
Assigned Definition.
@ param_assign
Parameter Variable.
NmodlType
NMODL variable properties.
@ pointer_var
Pointer Type.
@ bbcore_pointer_var
Bbcorepointer Type.
@ state_var
state variable
@ derivative_block
Derivative Block.
@ non_linear_block
NonLinear Block.
@ global_var
Global Variable.
std::vector< std::string > to_string_vector(const NmodlType &obj)
helper function to convert nmodl properties to string
@ random_var
Randomvar Type.
@ localized
converted to local
@ discrete_block
Discrete Block.
@ extern_neuron_variable
neuron variable accessible in mod file
@ electrode_cur_var
Electrode Current.
bool has_property(const NmodlType &obj, NmodlType property)
check if any property is set