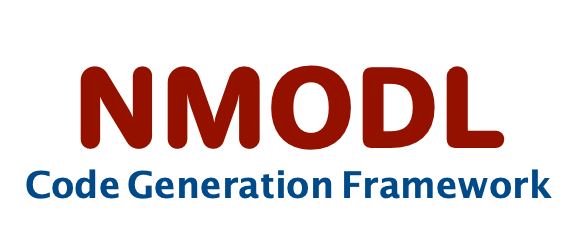 |
User Guide
|
Go to the documentation of this file.
8 #include <catch2/catch_test_macros.hpp>
9 #include <catch2/matchers/catch_matchers_string.hpp>
18 using Catch::Matchers::ContainsSubstring;
20 using namespace nmodl;
21 using namespace visitor;
22 using namespace codegen;
28 const std::string& simulator =
"coreneuron") {
30 SymtabVisitor().visit_program(*ast);
31 PerfVisitor().visit_program(*ast);
32 return CodegenCompatibilityVisitor(simulator).find_unhandled_ast_nodes(*ast);
35 SCENARIO(
"Uncompatible constructs should failed",
"[codegen][compatibility_visitor]") {
36 GIVEN(
"A mod file containing an EXTERNAL construct") {
37 std::string
const nmodl_text = R
"(
43 THEN("should fail for CoreNEURON") {
48 THEN(
"but succeed for NEURON") {
53 GIVEN(
"A mod file containing a written GLOBAL var") {
54 std::string
const nmodl_text = R
"(
64 THEN("should failed") {
69 GIVEN(
"A mod file containing a written un-writtable var") {
70 std::string
const nmodl_text = R
"(
80 THEN("should failed") {
85 GIVEN(
"A mod file with BBCOREPOINTER without bbcore_read / bbcore_write") {
86 std::string
const nmodl_text = R
"(
92 THEN("should failed") {
97 GIVEN(
"A mod file with BBCOREPOINTER without bbcore_write") {
98 std::string
const nmodl_text = R
"(
104 static void bbcore_read(double* x, int* d, int* xx, int* offset, _threadargsproto_) {
109 THEN("should failed") {
114 GIVEN(
"A mod file with BBCOREPOINTER without bbcore_read") {
115 std::string
const nmodl_text = R
"(
121 static void bbcore_write(double* x, int* d, int* xx, int* offset, _threadargsproto_) {
126 THEN("should failed") {
131 GIVEN(
"A mod file with BBCOREPOINTER with bbcore_read / bbcore_write") {
132 std::string
const nmodl_text = R
"(
138 static void bbcore_read(double* x, int* d, int* xx, int* offset, _threadargsproto_) {
140 static void bbcore_write(double* x, int* d, int* xx, int* offset, _threadargsproto_) {
145 THEN("should succeed") {
150 GIVEN(
"A mod file with a no SOLVE method") {
151 std::string
const nmodl_text = R
"(
157 THEN("should succeed") {
162 GIVEN(
"A mod file with a invalid SOLVE method") {
163 std::string
const nmodl_text = R
"(
165 SOLVE state METHOD runge
169 THEN("should failed") {
Class that binds all pieces together for parsing nmodl file.
SCENARIO("Uncompatible constructs should failed", "[codegen][compatibility_visitor]")
Visitor for measuring performance related information
encapsulates code generation backend implementations
bool runCompatibilityVisitor(const std::string &nmodl_text, const std::string &simulator="coreneuron")
Return true if it failed and false otherwise.
Auto generated AST classes declaration.
Visitor for printing compatibility issues of the mod file
std::shared_ptr< ast::Program > parse_string(const std::string &input)
parser nmodl provided as string (used for testing)
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.