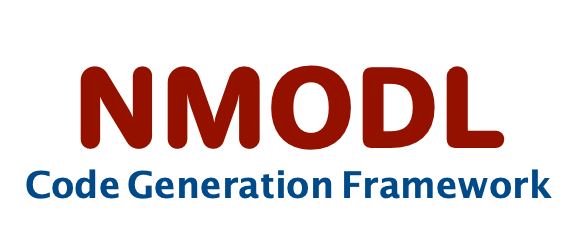 |
User Guide
|
Go to the documentation of this file.
17 #include <unordered_map>
82 std::shared_ptr<ast::Program>
astRoot =
nullptr;
89 std::unordered_map<std::string, const location*>
open_files;
110 std::shared_ptr<ast::Program>
parse_stream(std::istream& in);
113 std::shared_ptr<ast::Program>
parse_string(
const std::string& input);
121 std::shared_ptr<ast::Program>
parse_file(
const std::filesystem::path& filename,
122 const location* loc =
nullptr);
124 std::shared_ptr<ast::Include>
parse_include(
const std::filesystem::path& filename,
125 const location& loc);
136 const std::shared_ptr<ast::Program>&
get_ast() const noexcept {
149 void parse_error(
const location& location,
const std::string& message);
156 const location& location,
157 const std::string& message);
Class that binds all pieces together for parsing nmodl file.
void set_ast(ast::Program *node) noexcept
set new ast root
std::shared_ptr< ast::Include > parse_include(const std::filesystem::path &filename, const location &loc)
std::string stream_name
file or input stream name (used by scanner for position), see todo
const std::shared_ptr< ast::Program > & get_ast() const noexcept
return previously parsed AST otherwise nullptr
encapsulates code generation backend implementations
std::ostringstream parser_stream
The stream where Bison will dump its logs.
bool trace_scanner
enable debug output in the flex scanner
int get_defined_var_value(const std::string &name) const
return variable's value defined as macro (always an integer)
void add_defined_var(const std::string &name, int value)
add macro definition and it's value (DEFINE keyword of nmodl)
std::shared_ptr< ast::Program > parse_stream(std::istream &in)
parse nmodl file provided as istream
std::string check_include_argument(const location &location, const std::string &filename)
Ensure file argument given to the INCLUDE directive is valid:
std::shared_ptr< ast::Program > parse_file(const std::filesystem::path &filename, const location *loc=nullptr)
parse NMODL file
void parse_error(const location &location, const std::string &message)
Emit a parsing error.
Represent Lexer/Scanner class for NMODL language parsing.
Auto generated AST classes declaration.
bool trace_parser
enable debug output in the bison parser
bool is_defined_var(const std::string &name) const
check if particular text is defined as macro
bool is_verbose() const noexcept
static FileLibrary default_instance()
Initialize the library with the following path:
FileLibrary library
The file library for IMPORT directives.
std::shared_ptr< ast::Program > parse_string(const std::string &input)
parser nmodl provided as string (used for testing)
std::shared_ptr< ast::Program > astRoot
root of the ast
bool verbose
print messages from lexer/parser
std::unordered_map< std::string, int > defined_var
all macro defined in the mod file
Represents top level AST node for whole NMODL input.
std::unordered_map< std::string, const location * > open_files
The list of open files, and the location of the request.