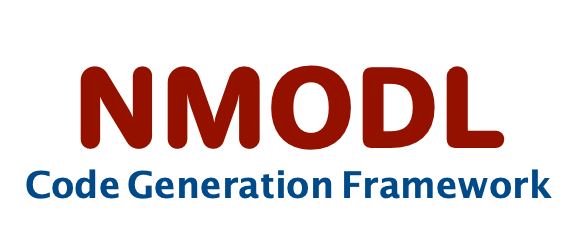 |
User Guide
|
Go to the documentation of this file.
16 #include "parser/nmodl/nmodl_parser.hpp"
24 #define YY_DECL nmodl::parser::NmodlParser::symbol_type nmodl::parser::NmodlLexer::next_token()
33 #ifndef __FLEX_LEXER_H
34 #define yyFlexLexer NmodlFlexLexer
35 #include "FlexLexer.h"
99 std::istream* in =
nullptr,
100 std::ostream* out =
nullptr)
101 : NmodlFlexLexer(in, out)
Class that binds all pieces together for parsing nmodl file.
~NmodlLexer() override=default
NmodlLexer(NmodlDriver &driver, std::istream *in=nullptr, std::ostream *out=nullptr)
NmodlLexer constructor.
ast::String * last_unit
Units are stored in the scanner (could be stored in the driver though)
location loc
location of the parsed token
encapsulates code generation backend implementations
ast::String * get_unit()
Return last scanned unit as ast::String.
void reset_end_position()
Reset the column position of lexer to 0.
void set_debug(bool b)
Enable debug output (via yyout) if compiled into the scanner.
int lexical_context
Context of the reaction (~) token.
Represent Lexer/Scanner class for NMODL language parsing.
Auto generated AST classes declaration.
virtual NmodlParser::symbol_type next_token()
Function for lexer to scan token (replacement for yylex())
std::string get_curr_line() const
Return current line as string.
NmodlDriver & driver
Reference to driver object where this lexer resides.
void symbol_type(const std::string &name, T &value)
std::string input_line()
Input text until end of line.
void scan_unit()
Scan subsequent text as unit.