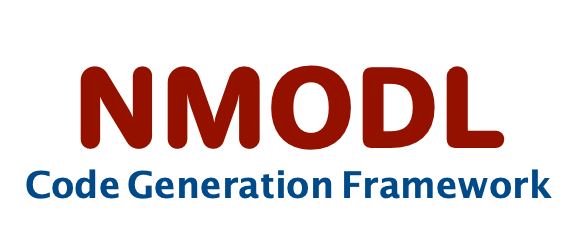 |
User Guide
|
Go to the documentation of this file.
11 #include <catch2/catch_test_macros.hpp>
26 using namespace nmodl;
33 std::istringstream ss(name);
34 std::istream& in = ss;
40 value = sym.value.as<T>();
43 TEST_CASE(
"NMODL Lexer returning valid ModToken object",
"[token][modtoken]") {
44 SECTION(
"test for ast::Name") {
50 REQUIRE(ss.str() ==
" text at [1.1-4] type 343");
57 REQUIRE(ss.str() ==
" some_text at [1.3-11] type 343");
61 SECTION(
"test for ast::Prime") {
67 REQUIRE(ss.str() ==
" h'' at [1.1-3] type 350");
73 TEST_CASE(
"Addition of two ModToken objects",
"[token][modtoken]") {
74 SECTION(
"adding two random strings") {
80 nmodl::parser::position adder1_begin(
nullptr, 1, 1);
81 nmodl::parser::position adder1_end(
nullptr, 1, 5);
83 ModToken adder1(
"text", 1, adder1_location);
85 nmodl::parser::position adder2_begin(
nullptr, 2, 1);
86 nmodl::parser::position adder2_end(
nullptr, 2, 5);
89 ModToken adder2(
"text", 2, adder2_location);
98 " text at [1.1-4] type 1 + text at [2.1-4] type 2 = "
99 " text at [1.1-2.4] type 1");
Class that binds all pieces together for parsing nmodl file.
nmodl::parser::location LocationType
TEST_CASE("NMODL Lexer returning valid ModToken object", "[token][modtoken]")
encapsulates code generation backend implementations
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
std::shared_ptr< Integer > get_order() const noexcept
Getter for member variable PrimeName::order.
nmodl::parser::UnitDriver driver
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
Represent Lexer/Scanner class for NMODL language parsing.
Represents a prime variable (for ODE)
virtual NmodlParser::symbol_type next_token()
Function for lexer to scan token (replacement for yylex())
void symbol_type(const std::string &name, T &value)
Represent token returned by scanner.