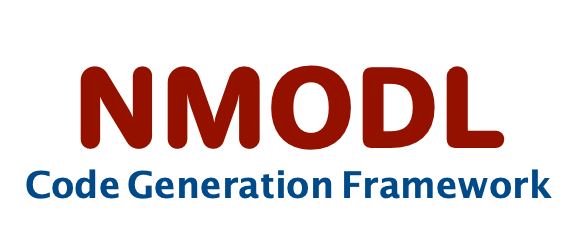 |
User Guide
|
Go to the documentation of this file.
8 #include <catch2/catch_test_macros.hpp>
16 using namespace nmodl;
17 using namespace visitor;
18 using namespace test_utils;
31 std::stringstream stream;
32 NmodlPrintVisitor(stream).visit_program(*ast);
37 SCENARIO(
"Adding a variable for a table inside a function",
"[visitor][transform]") {
38 GIVEN(
"A mod file with a table inside a function") {
40 std::string input_nmodl_text = R
"(
42 TABLE FROM 0 TO 150 WITH 1
48 std::string output_nmodl_text = R
"(
50 TABLE mAlpha FROM 0 TO 150 WITH 1
56 std::string expected_output = reindent_text(output_nmodl_text);
58 THEN("variable has been added") {
60 REQUIRE(result == expected_output);
Class that binds all pieces together for parsing nmodl file.
std::string reindent_text(const std::string &text, int indent_level)
Reindent nmodl text for text-to-text comparison.
encapsulates code generation backend implementations
void visit_program(ast::Program &node) override
visit node of type ast::Program
Auto generated AST classes declaration.
nmodl::parser::UnitDriver driver
bool parse_string(const std::string &input)
parser Units provided as string (used for testing)
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.