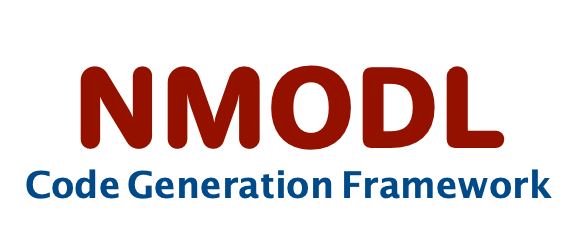 |
User Guide
|
Go to the documentation of this file.
52 std::shared_ptr<nmodl::units::UnitTable>
table = std::make_shared<nmodl::units::UnitTable>();
std::shared_ptr< nmodl::units::UnitTable > table
shared pointer to the UnitTable that stores all the unit definitions
UnitLexer * lexer
pointer to the lexer instance being used
encapsulates code generation backend implementations
std::string stream_name
file or input stream name (used by scanner for position), see todo
Classes for storing different units specification.
bool parse_string(const std::string &input)
parser Units provided as string (used for testing)
bool verbose
print messages from lexer/parser
Represent Lexer/Scanner class for Units parsing.
Class that binds all pieces together for parsing C units.
UnitParser * parser
pointer to the parser instance being used
bool parse_file(const std::string &filename)
parse Units file
bool parse_stream(std::istream &in)
parse Units file provided as istream