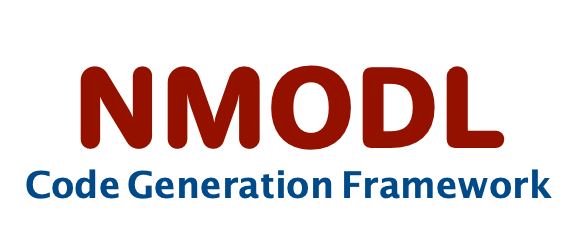 |
User Guide
|
Go to the documentation of this file.
10 #include "parser/unit/unit_parser.hpp"
17 #define YY_DECL nmodl::parser::UnitParser::symbol_type nmodl::parser::UnitLexer::next_token()
26 #ifndef __FLEX_LEXER_H
27 #define yyFlexLexer UnitFlexLexer
28 #include "FlexLexer.h"
65 std::istream* in =
nullptr,
66 std::ostream* out =
nullptr)
67 : UnitFlexLexer(in, out) {}
encapsulates code generation backend implementations
location loc
location of the parsed token
virtual UnitParser::symbol_type next_token()
Function for lexer to scan token (replacement for yylex())
UnitLexer(UnitDriver &, std::istream *in=nullptr, std::ostream *out=nullptr)
UnitLexer constructor.
void symbol_type(const std::string &name, T &value)
~UnitLexer() override=default
Represent Lexer/Scanner class for Units parsing.
Class that binds all pieces together for parsing C units.