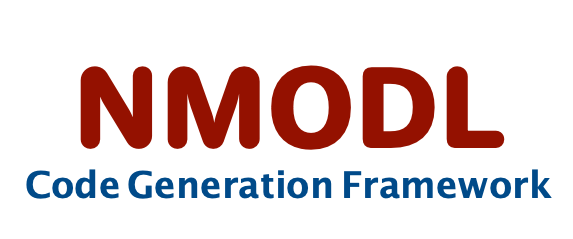 |
User Guide
|
Go to the documentation of this file.
17 #include <fmt/format.h>
23 #include <string_view>
45 std::streambuf*
sbuf =
nullptr;
46 std::unique_ptr<std::ostream>
result;
54 :
result(std::make_unique<std::ostream>(std::cout.rdbuf()))
58 :
result(std::make_unique<std::ostream>(stream.rdbuf()))
74 void push_block(
const std::string& expression);
79 template <
typename... Args>
85 template <
typename... Args>
88 add_text(std::forward<Args>(args)...);
93 template <
typename... Args>
95 add_line(fmt::format(std::forward<Args>(args)...));
99 template <
typename... Args>
101 push_block(fmt::format(std::forward<Args>(args)...));
105 template <
typename... Args>
107 add_text(fmt::format(std::forward<Args>(args)...));
130 void pop_block(
const std::string_view& suffix, std::size_t num_newlines = 1);
void add_indent()
print whitespaces for indentation
void add_multi_line(const std::string &)
void blame()
Blame when on the requested line.
void add_text(Args &&... args)
void pop_block_nl(std::size_t num_newlines=0)
same as pop_block but control the number of NL characters (0 or more) with num_newlines parameter
encapsulates code generation backend implementations
void fmt_text(Args &&... args)
fmt_text(args...) is just shorthand for add_text(fmt::format(args...))
CodePrinter(size_t blame_line=0)
std::unique_ptr< std::ostream > result
void add_line(Args &&... args)
void chain_block(std::string const &expression)
end a block and immediately start a new one (i.e. "[indent-1]} [expression] {\n")
CodePrinter(std::ostream &stream, size_t blame_line=0)
void pop_block()
end of current block scope (i.e. end with "}") and adds one NL character
std::ostream & operator<<(std::ostream &stream, const ModToken &mt)
void fmt_push_block(Args &&... args)
fmt_push_block(args...) is just shorthand for push_block(fmt::format(args...))
void fmt_line(Args &&... args)
fmt_line(x, y, z) is just shorthand for add_line(fmt::format(x, y, z))
void push_block()
start a block scope without indentation (i.e. "{\n")
void add_newline(std::size_t n=1)
Helper class for printing C/C++ code.