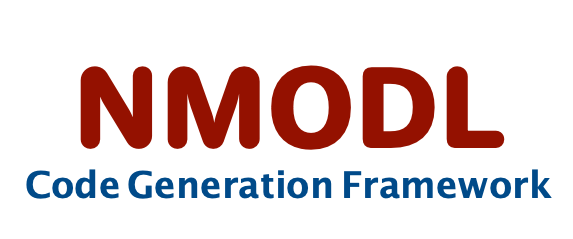 |
User Guide
|
Go to the documentation of this file.
28 stream << std::setw(15) << mt.
name <<
" at [" << mt.
position() <<
"]";
29 return stream <<
" type " << mt.
token;
int token
token value returned by lexer
nmodl::parser::location LocationType
encapsulates code generation backend implementations
std::string name
name of the token
std::string position() const
Return position of the token as string.
bool external
true if token is externally defined variable (e.g. t, dt in NEURON)
ModToken operator+(ModToken const &adder1, ModToken const &adder2)
LocationType pos
position of token in the mod file
std::ostream & operator<<(std::ostream &stream, const ModToken &mt)
int start_line() const
Return line number where token started in the mod file.
Represent token returned by scanner.