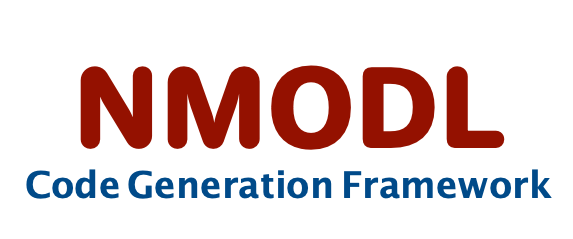 |
User Guide
|
Go to the documentation of this file.
15 #include "parser/nmodl/location.hh"
101 return pos.begin.line;
106 return pos.begin.column;
friend std::ostream & operator<<(std::ostream &stream, const ModToken &mt)
Overload << operator to print object.
int token
token value returned by lexer
int type() const
Return token type from lexer.
encapsulates code generation backend implementations
friend ModToken operator+(ModToken const &adder1, ModToken const &adder2)
Overload + operator to create an object whose position starts from the start line and column of the f...
std::string name
name of the token
std::string position() const
Return position of the token as string.
ModToken(std::string name, int token, LocationType &pos)
bool external
true if token is externally defined variable (e.g. t, dt in NEURON)
LocationType pos
position of token in the mod file
std::string text() const
Return token text from mod file.
int start_line() const
Return line number where token started in the mod file.
ModToken * clone() const
Return a new instance of token.
int start_column() const
Return start of column number where token appear in the mod file.
Represent token returned by scanner.
nmodl::parser::location LocationType