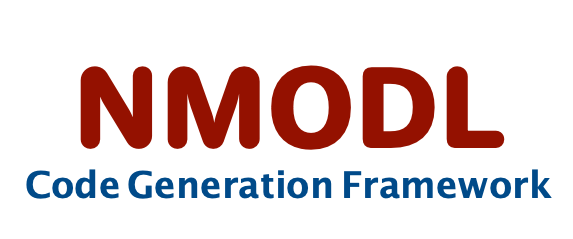 |
User Guide
|
Go to the documentation of this file.
16 #include <unordered_set>
39 std::pair<std::string, std::unordered_set<std::string>>
dependencies;
std::string get_indexed_name()
get the attribute indexed_name
Auto generated AST classes declaration.
void visit_indexed_name(ast::IndexedName &node) override
Get node name with index for the IndexedName node.
Represents differential equation in DERIVATIVE block.
encapsulates code generation backend implementations
void visit_program(ast::Program &node) override
visit node of type ast::Program
Auto generated AST classes declaration.
Auto generated AST classes declaration.
Represents specific element of an array variable.
std::pair< std::string, std::unordered_set< std::string > > get_dependencies()
get the attribute dependencies
Concrete visitor for all AST classes.
IndexedNameVisitor()=default
Default constructor.
Get node name with indexed for the IndexedName node and the dependencies of DiffEqExpression node.
std::pair< std::string, std::unordered_set< std::string > > dependencies
Represents top level AST node for whole NMODL input.
void visit_diff_eq_expression(ast::DiffEqExpression &node) override
Get dependencies for the DiffEqExpression node.
Concrete visitor for all AST classes.