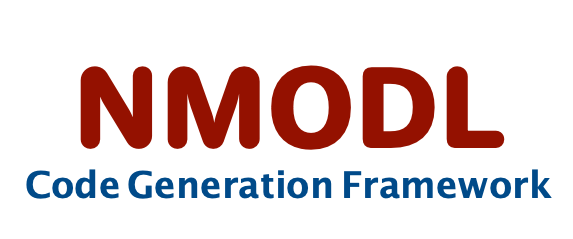 |
User Guide
|
Go to the documentation of this file.
51 std::shared_ptr<Identifier>
name;
55 std::shared_ptr<ModToken>
token;
119 return "IndexedName";
127 return std::static_pointer_cast<IndexedName>(shared_from_this());
134 return std::static_pointer_cast<const IndexedName>(shared_from_this());
167 std::shared_ptr<Identifier>
get_name() const noexcept {
std::shared_ptr< Expression > get_length() const noexcept
Getter for member variable IndexedName::length.
@ INDEXED_NAME
type of ast::IndexedName
Abstract base class for all constant visitors implementation.
Base class for all identifiers.
void set_parent_in_children()
Set this object as parent for all the children.
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
std::string get_node_name() const override
Return name of the node.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
void set_name(std::shared_ptr< Identifier > &&name)
Setter for member variable IndexedName::name (rvalue reference)
std::shared_ptr< Identifier > get_name() const noexcept
Getter for member variable IndexedName::name.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
Abstract Syntax Tree (AST) related implementations.
AstNodeType
Enum type for every AST node type.
IndexedName * clone() const override
Return a copy of the current node.
Represents specific element of an array variable.
Abstract base class for all visitors implementation.
std::shared_ptr< Identifier > name
Name of array variable.
std::shared_ptr< ModToken > token
token with location information
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
void set_token(const ModToken &tok)
Set token for the current ast node.
virtual ~IndexedName()=default
void visit_children(visitor::Visitor &v) override
visit children i.e.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
std::shared_ptr< Expression > length
length of an array or index position
bool is_indexed_name() const noexcept override
Check if the ast node is an instance of ast::IndexedName.
Auto generated AST classes declaration.
Base class for all expressions in the NMODL.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
IndexedName(Identifier *name, Expression *length)
Represent token returned by scanner.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
void set_length(std::shared_ptr< Expression > &&length)
Setter for member variable IndexedName::length (rvalue reference)