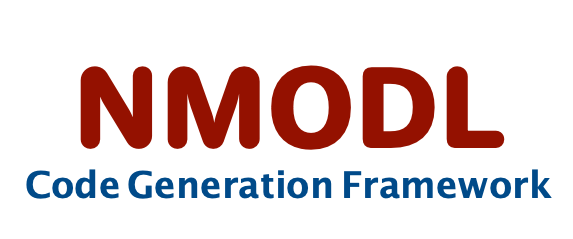 |
User Guide
|
Go to the documentation of this file.
151 std::map<std::shared_ptr<ast::Statement>,
152 std::vector<std::shared_ptr<ast::ExpressionStatement>>>
void inline_arguments(ast::StatementBlock &inlined_block, const ast::ArgumentVector &callee_parameters, const ast::ExpressionVector &caller_expressions)
add assignment statements into given statement block to inline arguments
std::map< std::shared_ptr< ast::Statement >, std::vector< std::shared_ptr< ast::ExpressionStatement > > > inlined_statements
map to track statements being prepended before function call (typically for function calls)
std::map< std::string, int > inlined_variables
variables currently being renamed and their count (for renaming)
encapsulates code generation backend implementations
ast::StatementBlock * caller_block
statement block containing current function call
bool can_replace_statement(const std::shared_ptr< ast::Statement > &statement)
true if statement can be replaced with inlined body this is possible for standalone function/procedur...
std::stack< ast::StatementBlock * > statementblock_stack
statement blocks in call hierarchy
bool inline_function_call(ast::Block &callee, ast::FunctionCall &node, ast::StatementBlock &caller)
inline function/procedure into caller block
static bool can_inline_block(const ast::StatementBlock &block)
true if given statement block can be inlined
Base class for all block scoped nodes.
std::map< std::shared_ptr< ast::Statement >, ast::ExpressionStatement * > replaced_statements
map to track the statements being replaces (typically for procedure calls)
void visit_function_call(ast::FunctionCall &node) override
visit node of type ast::FunctionCall
std::vector< std::shared_ptr< Argument > > ArgumentVector
Visitor to inline local procedure and function calls
void visit_program(ast::Program &node) override
visit node of type ast::Program
Concrete visitor for all AST classes.
std::stack< std::shared_ptr< ast::Statement > > statement_stack
statements being executed in call hierarchy
Represent symbol table for a NMODL block.
Represents block encapsulating list of statements.
std::vector< std::shared_ptr< Expression > > ExpressionVector
static void add_return_variable(ast::StatementBlock &block, std::string &varname)
add assignment statement at end of block (to use as a return statement in case of procedure blocks)
void visit_wrapped_expression(ast::WrappedExpression &node) override
Visit all wrapped expressions which can contain function calls.
symtab::SymbolTable const * program_symtab
symbol table for program node
std::shared_ptr< ast::Statement > caller_statement
statement containing current function call
std::map< ast::FunctionCall *, std::string > replaced_fun_calls
map to track replaced function calls (typically for function calls)
Represents top level AST node for whole NMODL input.
Forward declarations of symbols in namespace nmodl::symtab.
Wrap any other expression type.
void visit_statement_block(ast::StatementBlock &node) override
visit node of type ast::StatementBlock
Concrete visitor for all AST classes.