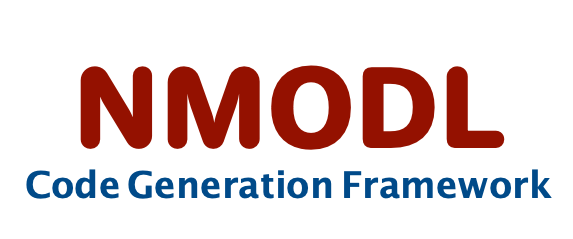 |
User Guide
|
Go to the documentation of this file.
31 logger->debug(
"KineticBlockVisitor :: adding non-state fflux[{}] \"{}\"",
36 logger->debug(
"KineticBlockVisitor :: adding non-state bflux[{}] \"{}\"",
44 int i_statevar = it->second;
48 logger->debug(
"KineticBlockVisitor :: nu_L[{}][{}] += {}",
55 logger->debug(
"KineticBlockVisitor :: nu_R[{}][{}] += {}",
76 if (compartment_factor.empty()) {
88 "KineticBlockVisitor :: Error : CONSERVE statement should only contain state vars "
89 "on LHS, but found {}",
96 std::shared_ptr<ast::Expression>
create_expr(
const std::string& str_expr) {
98 auto expr = std::dynamic_pointer_cast<ast::ExpressionStatement>(statement)->get_expression();
99 return std::dynamic_pointer_cast<ast::BinaryExpression>(expr)->get_rhs();
110 logger->debug(
"KineticBlockVisitor :: CONSERVE statement: {}",
to_nmodl(node));
135 auto expr = std::dynamic_pointer_cast<ast::Conserve>(statement);
149 std::string expression =
to_nmodl(expr);
150 logger->debug(
"KineticBlockVisitor :: COMPARTMENT expr: {}", expression);
151 for (
const auto& name_ptr: node.
get_names()) {
152 const auto& var_name = name_ptr->get_node_name();
155 int var_index = it->second;
158 "KineticBlockVisitor :: COMPARTMENT factor {} for state var {} (index {})",
207 logger->debug(
"KineticBlockVisitor :: '<<' reaction statement: {}",
to_nmodl(node));
217 bool single_state_var =
true;
218 if (lhs->is_react_var_name()) {
219 auto value = std::dynamic_pointer_cast<ast::ReactVarName>(lhs)->get_value();
220 if (value && (value->eval() != 1)) {
221 single_state_var =
false;
224 if (!lhs->is_react_var_name() || !single_state_var) {
226 "KineticBlockVisitor :: LHS of \"<<\" reaction statement must be a single state "
227 "var, but instead found {}: ignoring this statement",
232 std::string varname =
to_nmodl(lhs);
236 int var_index = it->second;
243 logger->debug(
"KineticBlockVisitor :: '<<' reaction statement: {}' += {}",
290 std::string multiply_var = std::string(
"*").append(
state_var[j]);
331 auto var_name = std::dynamic_pointer_cast<ast::Name>(node.
get_expression());
332 if (var_name->get_node_name() ==
"f_flux") {
334 logger->debug(
"KineticBlockVisitor :: replacing f_flux with {}",
to_nmodl(expr));
336 }
else if (var_name->get_node_name() ==
"b_flux") {
338 logger->debug(
"KineticBlockVisitor :: replacing b_flux with {}",
to_nmodl(expr));
380 for (
int j = 0; j < Nj; ++j) {
383 for (
int i = 0; i < Ni; ++i) {
387 if (!ode_rhs.empty()) {
390 if (
bflux[i].empty()) {
391 ode_rhs += fmt::format(
"({}*({}))", delta_nu,
fflux[i]);
392 }
else if (
fflux[i].empty()) {
393 ode_rhs += fmt::format(
"({}*(-{}))", delta_nu,
bflux[i]);
395 ode_rhs += fmt::format(
"({}*({}-{}))", delta_nu,
fflux[i],
bflux[i]);
404 if (!ode_rhs.empty()) {
406 std::string var_str = state_var_split[0];
407 std::string index_str;
408 if (state_var_split.size() > 1) {
409 index_str =
"[" + state_var_split[1];
411 odes.push_back(fmt::format(
"{}'{} = {}", var_str, index_str, ode_rhs));
415 for (
const auto& ode:
odes) {
416 logger->debug(
"KineticBlockVisitor :: ode : {}", ode);
423 for (
const auto& ode:
odes) {
424 logger->debug(
"KineticBlockVisitor :: -> adding statement: {}", ode);
443 auto statevars = symtab->get_variables_with_properties(NmodlType::state_var);
444 for (
const auto& v: statevars) {
445 std::string var_name = v->get_name();
451 for (
int i = 0; i < v->get_length(); ++i) {
453 logger->debug(
"KineticBlockVisitor :: state_var_index[{}] = {}",
460 logger->debug(
"KineticBlockVisitor :: state_var_index[{}] = {}",
471 for (
const auto& ii: kineticBlockNodes) {
478 for (
auto it = blocks.begin(); it != blocks.end(); ++it) {
479 if (it->get() == kinetic_block) {
481 std::make_shared<ast::DerivativeBlock>(kinetic_block->get_name(),
482 kinetic_block->get_statement_block());
484 dblock->set_token(tok);
void visit_wrapped_expression(ast::WrappedExpression &node) override
visit node of type ast::WrappedExpression
std::string to_nmodl(const ast::Ast &node, const std::set< ast::AstNodeType > &exclude_types)
Given AST node, return the NMODL string representation.
std::shared_ptr< Expression > get_expression() const noexcept
Getter for member variable WrappedExpression::expression.
std::shared_ptr< Expression > get_expression1() const noexcept
Getter for member variable ReactionStatement::expression1.
std::unordered_map< std::string, int > array_state_var_size
unordered_map from array state variable to its size (for summing over each element of any array state...
Implement class to represent a symbol in Symbol Table.
ReactionOp get_value() const noexcept
Getter for member variable ReactionOperator::value.
@ KINETIC_BLOCK
type of ast::KineticBlock
std::shared_ptr< Expression > get_expression() const noexcept
Getter for member variable Compartment::expression.
void visit_statement_block(ast::StatementBlock &node) override
visit node of type ast::StatementBlock
void process_conserve_reac_var(const std::string &varname, int count=1)
update CONSERVE statement with reaction var term
void visit_children(visitor::Visitor &v) override
visit children i.e.
Represent CONSERVE statement in NMODL.
std::shared_ptr< Expression > get_expr() const noexcept
Getter for member variable Conserve::expr.
std::shared_ptr< Expression > get_expression2() const noexcept
Getter for member variable ReactionStatement::expression2.
std::vector< std::string > non_state_var_fflux
multiplicate constant terms for fluxes from non-state vars as reactants e.g.
int conserve_statement_count
counts the number of CONSERVE statements in Kinetic blocks
std::shared_ptr< StatementBlock > get_statement_block() const noexcept override
Getter for member variable KineticBlock::statement_block.
encapsulates code generation backend implementations
std::vector< std::string > compartment_factors
multiplicative factors for ODEs from COMPARTMENT statements
void visit_program(ast::Program &node) override
visit node of type ast::Program
int state_var_count
number of state variables
void visit_compartment(ast::Compartment &node) override
visit node of type ast::Compartment
std::shared_ptr< VarName > get_name() const noexcept
Getter for member variable ReactVarName::name.
Implement string manipulation functions.
std::vector< std::string > fflux
generated set of fluxes and ODEs
std::string conserve_equation_statevar
conserve statement: current state variable being processed
void set_expr(std::shared_ptr< Expression > &&expr)
Setter for member variable Conserve::expr (rvalue reference)
void visit_reaction_operator(ast::ReactionOperator &node) override
visit node of type ast::ReactionOperator
void visit_children(visitor::Visitor &v) override
visit children i.e.
const NameVector & get_names() const noexcept
Getter for member variable Compartment::names.
std::shared_ptr< Expression > get_reaction1() const noexcept
Getter for member variable ReactionStatement::reaction1.
struct nmodl::visitor::KineticBlockVisitor::RateEqs rate_eqs
void visit_conserve(ast::Conserve &node) override
visit node of type ast::Conserve
Visitor for kinetic block statements
Utility functions for visitors implementation.
bool in_reaction_statement_lhs
true if we are visiting the left hand side of reaction statement
int i_statement
current statement index
StatementVector::const_iterator erase_statement(StatementVector::const_iterator first)
Erase member to statements.
void visit_children(visitor::Visitor &v) override
visit children i.e.
bool in_conserve_statement
true if we are visiting a CONSERVE statement
std::unordered_set< ast::Statement * > statements_to_remove
statements to remove from block
std::string to_string(const T &obj)
void set_expression(std::shared_ptr< Expression > &&expression)
Setter for member variable WrappedExpression::expression (rvalue reference)
std::string modfile_fflux
current expressions for the fflux, bflux variables that can be used in the mod file and that are dete...
void visit_reaction_statement(ast::ReactionStatement &node) override
visit node of type ast::ReactionStatement
const ReactionOperator & get_op() const noexcept
Getter for member variable ReactionStatement::op.
std::vector< std::string > state_var
state variables vector
std::string conserve_equation_str
conserve statement equation as string
std::vector< std::string > bflux
std::shared_ptr< Statement > create_statement(const std::string &code_statement)
Convert given code statement (in string format) to corresponding ast node.
std::vector< std::shared_ptr< const ast::Ast > > collect_nodes(const ast::Ast &node, const std::vector< ast::AstNodeType > &types)
traverse node recursively and collect nodes of given types
Represent COMPARTMENT statement in NMODL.
std::shared_ptr< ast::Expression > create_expr(const std::string &str_expr)
void visit_react_var_name(ast::ReactVarName &node) override
visit node of type ast::ReactVarName
void visit_children(visitor::Visitor &v) override
visit children i.e.
static std::vector< std::string > split_string(const std::string &text, char delimiter)
Split a text in a list of words, using a given delimiter character.
Represents block encapsulating list of statements.
void set_react(std::shared_ptr< Expression > &&react)
Setter for member variable Conserve::react (rvalue reference)
NmodlType
NMODL variable properties.
const NodeVector & get_blocks() const noexcept
Getter for member variable Program::blocks.
std::string modfile_bflux
std::vector< std::string > k_b
std::vector< std::string > additive_terms
additive constant terms for ODEs from reaction statements like ~ x << (a)
void visit_kinetic_block(ast::KineticBlock &node) override
visit node of type ast::KineticBlock
std::unordered_map< std::string, int > state_var_index
unordered_map from state variable to corresponding index
Implement logger based on spdlog library.
std::vector< ast::KineticBlock * > kinetic_blocks
vector of kinetic block nodes
ast::StatementBlock * current_statement_block
current statement block being visited
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
std::vector< std::string > k_f
std::vector< std::string > odes
void process_reac_var(const std::string &varname, int count=1)
update stoichiometric matrices with reaction var term
Represents top level AST node for whole NMODL input.
void visit_children(visitor::Visitor &v) override
visit children i.e.
bool in_reaction_statement
true if we are visiting a reaction statement
void set_blocks(NodeVector &&blocks)
Setter for member variable Program::blocks (rvalue reference)
std::vector< std::vector< int > > nu_R
std::shared_ptr< Integer > get_value() const noexcept
Getter for member variable ReactVarName::value.
Represent token returned by scanner.
std::vector< std::vector< int > > nu_L
Auto generated AST classes declaration.
Wrap any other expression type.
std::vector< std::string > non_state_var_bflux
std::string conserve_equation_factor
conserve statement: current state var multiplicative factor being processed