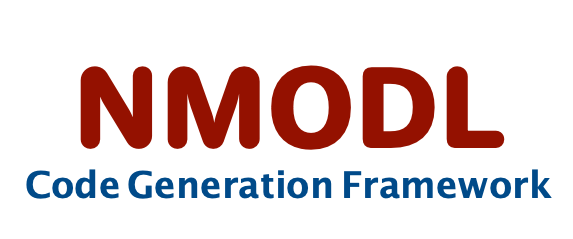 |
User Guide
|
Go to the documentation of this file.
20 using symtab::SymbolTable;
30 if (current_symtab !=
nullptr) {
41 item->visit_children(*
this);
57 if (parent_symtab ==
nullptr || variables ==
nullptr) {
63 for (
const auto& var: variables->get_variables()) {
64 std::string name = var->get_node_name();
67 if (s && s->is_variable()) {
69 rename_visitor.
set(name, new_name);
72 symbol->set_name(new_name);
73 symbol->mark_renamed();
Auto generated AST classes declaration.
SymbolTable * get_parent_table() const noexcept
void visit_statement_block(const ast::StatementBlock &node) override
visit node of type ast::StatementBlock
std::shared_ptr< ast::LocalListStatement > get_local_list_statement(const StatementBlock &node)
Return pointer to local statement in the given block, otherwise nullptr.
encapsulates code generation backend implementations
Auto generated AST classes declaration.
const StatementVector & get_statements() const noexcept
Getter for member variable StatementBlock::statements.
Utility functions for visitors implementation.
const symtab::SymbolTable * symtab
non-null symbol table in the scope hierarchy
Auto generated AST classes declaration.
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
Visitor to rename local variables conflicting with global scope
Blindly rename given variable to new name
Represent symbol table for a NMODL block.
void set(const std::string &old_name, std::string new_name)
std::map< std::string, int > renamed_variables
variables currently being renamed and their count
std::stack< const symtab::SymbolTable * > symtab_stack
symbol tables in case of nested blocks
std::string get_new_name(const std::string &name, const std::string &suffix, std::map< std::string, int > &variables)
Return new name variable by appending _suffix_COUNT where COUNT is number of times the given variable...
Represents block encapsulating list of statements.
Blindly rename given variable to new name
void visit_statement_block(ast::StatementBlock &node) override
rename name conflicting variables in the statement block and it's all children
std::shared_ptr< Symbol > lookup_in_scope(const std::string &name) const
check if symbol with given name exist in the current table (including all parents)