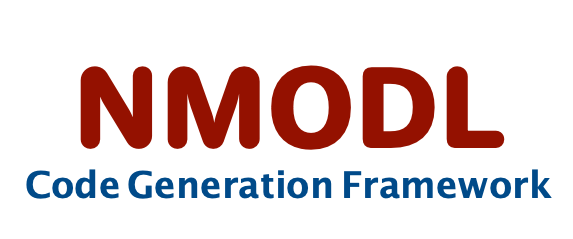 |
User Guide
|
encapsulates code generation backend implementations
const symtab::SymbolTable * symtab
non-null symbol table in the scope hierarchy
LocalVarRenameVisitor()=default
Concrete visitor for all AST classes.
Represent symbol table for a NMODL block.
std::map< std::string, int > renamed_variables
variables currently being renamed and their count
std::stack< const symtab::SymbolTable * > symtab_stack
symbol tables in case of nested blocks
Represents block encapsulating list of statements.
void visit_statement_block(ast::StatementBlock &node) override
rename name conflicting variables in the statement block and it's all children
Visitor to rename local variables conflicting with global scope
Forward declarations of symbols in namespace nmodl::symtab.
Concrete visitor for all AST classes.