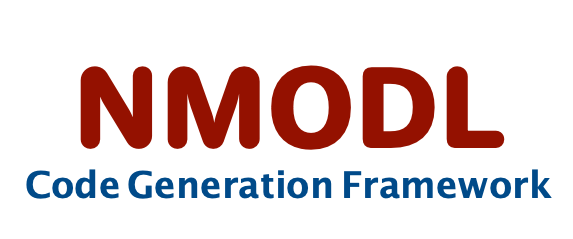 |
User Guide
|
Go to the documentation of this file.
53 std::string token_string = node.
get_token() !=
nullptr
56 logger->debug(
"RenameVisitor :: Renaming variable {}{} to {}",
81 const auto& text = statement->eval();
85 auto tokens =
driver.all_tokens();
87 std::ostringstream result;
88 for (
auto& token: tokens) {
94 logger->debug(
"RenameVisitor :: Renaming variable {} in VERBATIM block to {}",
101 statement->set(result.str());
bool add_prefix
add prefix to variable name
Represents a C code block.
std::shared_ptr< String > get_value() const noexcept
Getter for member variable Name::value.
encapsulates code generation backend implementations
void visit_name(const ast::Name &node) override
rename matching variable
void visit_prime_name(const ast::PrimeName &node) override
Prime name has member order which is an integer.
std::shared_ptr< String > get_statement() const noexcept
Getter for member variable Verbatim::statement.
Class that binds all pieces together for parsing C verbatim blocks.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.
std::string position() const
Return position of the token as string.
void visit_children(visitor::Visitor &v) override
visit children i.e.
Utility functions for visitors implementation.
nmodl::parser::UnitDriver driver
bool rename_verbatim
rename verbatim blocks as well
std::string new_var_name
new name
bool add_random_suffix
add random suffix
std::unordered_map< std::string, std::string > renamed_variables
Map that keeps the renamed variables to keep the same random suffix when a variable is renamed across...
Represents a prime variable (for ODE)
std::string new_var_name_prefix
variable prefix
std::string get_node_name() const override
Return name of the node.
Implement logger based on spdlog library.
std::string new_name_generator(const std::string &old_name)
Check if variable is already renamed and use the same naming otherwise add the new_name to the rename...
Blindly rename given variable to new name
std::string suffix_random_string(const std::set< std::string > &vars, const std::string &original_string, const UseNumbersInString use_num)
Return the "original_string" with a random suffix if "original_string" exists in "vars".
std::regex var_name_regex
regex for searching which variables to replace
void visit_verbatim(const ast::Verbatim &node) override
Parse verbatim blocks and rename variable if it is used.
std::set< std::string > get_global_vars(const Program &node)
Return set of strings with the names of all global variables.
Auto generated AST classes declaration.
std::shared_ptr< ast::Program > ast
ast::Ast* node