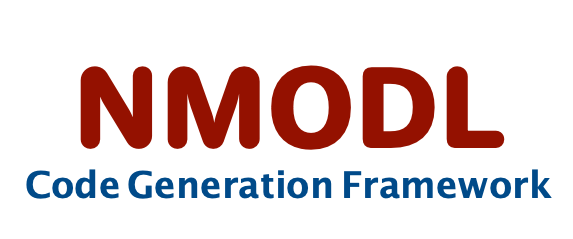 |
User Guide
|
SemanticAnalysisVisitor(bool accel_backend=false)
Concrete constant visitor for all AST classes.
bool in_mutex
true if we are inside a mutex locked part
bool in_function
true if we are in a function block
bool accel_backend
true if accelerator backend is used for code generation
encapsulates code generation backend implementations
void visit_procedure_block(const ast::ProcedureBlock &node) override
Store if we are in a procedure and if the arity of this is 1.
Represent MUTEXLOCK statement in NMODL.
Represents TABLE statement in NMODL.
void visit_name(const ast::Name &node) override
Only use of random_var is as first arg in random function.
void visit_table_statement(const ast::TableStatement &node) override
Visit a table statement and check that the arity of the block were 1.
symtab::SymbolTable * program_symtab
void visit_function_call(const ast::FunctionCall &node) override
random function first arg must be random_var
bool check(const ast::Program &node)
bool in_procedure
true if we are in a procedure block
Represents a DESTRUCTOR block in the NMODL.
void visit_function_block(const ast::FunctionBlock &node) override
Store if we are in a function and if the arity of this is 1.
Represent MUTEXUNLOCK statement in NMODL.
void visit_independent_block(const ast::IndependentBlock &node) override
Visit independent block and check if one of the variable is not t.
void visit_mutex_unlock(const ast::MutexUnlock &node) override
Look if MUTEXUNLOCK is outside a locked block.
void visit_program(const ast::Program &node) override
Check number of DERIVATIVE blocks.
Represent symbol table for a NMODL block.
void visit_protect_statement(const ast::ProtectStatement &node) override
Look if protect is inside a locked block.
Auto generated AST classes declaration.
void visit_function_table_block(const ast::FunctionTableBlock &node) override
Visit function table to check that number of args > 0.
bool one_arg_in_procedure_function
true if the procedure or the function contains only one argument
bool is_point_process
true if the mod file is of type point process
Represents a INDEPENDENT block in the NMODL.
void visit_destructor_block(const ast::DestructorBlock &node) override
Visit destructor and check that the file is of type POINT_PROCESS or ARTIFICIAL_CELL.
Represents top level AST node for whole NMODL input.
void visit_mutex_lock(const ast::MutexLock &node) override
Look if MUTEXLOCK is inside a locked block.
Concrete visitor for all AST classes.