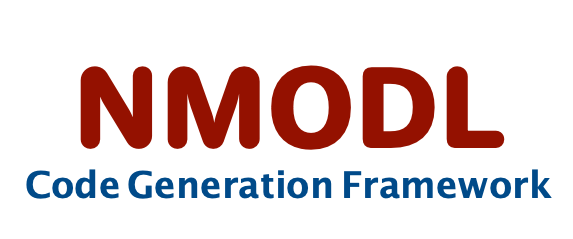 |
User Guide
|
Go to the documentation of this file.
42 std::shared_ptr<Name>
name;
46 std::shared_ptr<Unit>
unit;
50 std::shared_ptr<ModToken>
token;
116 return "ProcedureBlock";
138 return std::static_pointer_cast<ProcedureBlock>(shared_from_this());
145 return std::static_pointer_cast<const ProcedureBlock>(shared_from_this());
Abstract base class for all constant visitors implementation.
void set_statement_block(std::shared_ptr< StatementBlock > &&statement_block)
Setter for member variable ProcedureBlock::statement_block (rvalue reference)
const ArgumentVector & get_parameters() const noexcept override
Getter for member variable ProcedureBlock::parameters.
std::shared_ptr< ModToken > token
token with location information
std::string get_nmodl_name() const noexcept override
Return NMODL statement of ast node as std::string.
THIS FILE IS GENERATED AT BUILD TIME AND SHALL NOT BE EDITED.
ProcedureBlock(Name *name, const ArgumentVector ¶meters, Unit *unit, StatementBlock *statement_block)
void set_parameters(ArgumentVector &¶meters)
Setter for member variable ProcedureBlock::parameters (rvalue reference)
Auto generated AST classes declaration.
std::shared_ptr< Unit > unit
Unit if specified.
Abstract Syntax Tree (AST) related implementations.
void visit_children(visitor::Visitor &v) override
visit children i.e.
AstNodeType get_node_type() const noexcept override
Return type (ast::AstNodeType) of ast node.
void set_name(std::shared_ptr< Name > &&name)
Setter for member variable ProcedureBlock::name (rvalue reference)
std::shared_ptr< StatementBlock > get_statement_block() const noexcept override
Getter for member variable ProcedureBlock::statement_block.
AstNodeType
Enum type for every AST node type.
@ PROCEDURE_BLOCK
type of ast::ProcedureBlock
ArgumentVector parameters
Vector of the parameters.
Base class for all block scoped nodes.
std::shared_ptr< Name > get_name() const noexcept
Getter for member variable ProcedureBlock::name.
symtab::SymbolTable * symtab
symbol table for a block
std::vector< std::shared_ptr< Argument > > ArgumentVector
void accept(visitor::Visitor &v) override
accept (or visit) the current AST node using provided visitor
Abstract base class for all visitors implementation.
std::shared_ptr< Name > name
Name of the procedure.
virtual ~ProcedureBlock()=default
ProcedureBlock * clone() const override
Return a copy of the current node.
bool is_procedure_block() const noexcept override
Check if the ast node is an instance of ast::ProcedureBlock.
void set_unit(std::shared_ptr< Unit > &&unit)
Setter for member variable ProcedureBlock::unit (rvalue reference)
Represent symbol table for a NMODL block.
std::shared_ptr< Unit > get_unit() const noexcept
Getter for member variable ProcedureBlock::unit.
symtab::SymbolTable * get_symbol_table() const override
Return associated symbol table for the current ast node.
Represents block encapsulating list of statements.
void set_parent_in_children()
Set this object as parent for all the children.
std::string get_node_type_name() const noexcept override
Return type (ast::AstNodeType) of ast node as std::string.
Auto generated AST classes declaration.
std::shared_ptr< StatementBlock > statement_block
Block with statements vector.
void set_symbol_table(symtab::SymbolTable *newsymtab) override
Set symbol table for the current ast node.
Represent token returned by scanner.
std::shared_ptr< Ast > get_shared_ptr() override
Get std::shared_ptr from this pointer of the current ast node.
std::string get_node_name() const override
Return name of the node.
std::shared_ptr< const Ast > get_shared_ptr() const override
Get std::shared_ptr from this pointer of the current ast node.
void set_token(const ModToken &tok)
Set token for the current ast node.
const ModToken * get_token() const noexcept override
Return associated token for the current ast node.