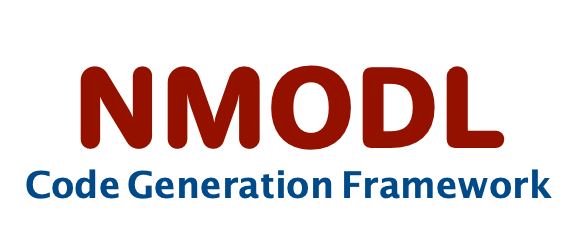 |
User Guide
|
Go to the documentation of this file.
10 #include "parser/c/c11_parser.hpp"
17 #define YY_DECL nmodl::parser::CParser::symbol_type nmodl::parser::CLexer::next_token()
26 #ifndef __FLEX_LEXER_H
27 #define yyFlexLexer CFlexLexer
28 #include "FlexLexer.h"
Represent Lexer/Scanner class for C (11) language parsing.
~CLexer() override=default
CLexer(CDriver &driver, std::istream *in=nullptr, std::ostream *out=nullptr)
CLexer constructor.
CParser::symbol_type get_token_type()
Get the type of token just parsed.
encapsulates code generation backend implementations
location loc
location of the parsed token
Class that binds all pieces together for parsing C verbatim blocks.
CDriver & driver
Reference to driver object which contains this lexer instance.
void symbol_type(const std::string &name, T &value)
virtual CParser::symbol_type next_token()
Function for lexer to scan token (replacement for yylex())