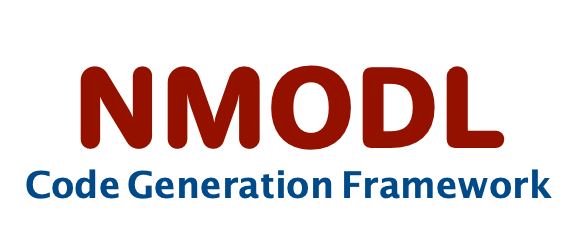 |
User Guide
|
Go to the documentation of this file.
32 using CodegenCoreneuronCppVisitor::CodegenCoreneuronCppVisitor;
104 const std::vector<std::string>& ptr_members)
override;
void print_net_send_buf_count_update_to_host() const override
update NetSendBuffer_t count from device to host
void print_device_stream_wait() const override
Print the code to synchronise/wait on stream specific to NrnThread.
void print_kernel_data_present_annotation_block_begin() override
annotations like "acc enter data present(...)" for main kernel
void print_parallel_iteration_hint(BlockType type, const ast::Block *block) override
ivdep like annotation for channel iterations
void print_net_send_buffering_grow() override
Replace default implementation by a no-op since the buffer cannot be grown up during gpu execution.
void print_newtonspace_transfer_to_device() const override
transfer newtonspace structure to device
Visitor for printing C++ code compatible with legacy api of CoreNEURON
void print_nrn_cur_matrix_shadow_reduction() override
reduction to matrix elements from shadow vectors
void print_net_send_buffering_cnt_update() const override
print atomic update of NetSendBuffer_t cnt
void print_net_init_acc_serial_annotation_block_begin() override
start of annotation "acc kernels" for net_init kernel
void print_deriv_advance_flag_transfer_to_device() const override
update derivimplicit advance flag on the gpu device
bool nrn_cur_reduction_loop_required() override
if reduction block in nrn_cur required
encapsulates code generation backend implementations
void print_device_atomic_capture_annotation() const override
print atomic capture pragma
void print_fast_imem_calculation() override
fast membrane current calculation
void print_atomic_reduction_pragma() override
atomic update pragma for reduction statements
Base class for all block scoped nodes.
void print_instance_struct_transfer_routine_declarations() override
declare helper functions for copying the instance struct to the device
Visitor for printing C++ code compatible with legacy api of CoreNEURON
void print_nrn_cur_matrix_shadow_update() override
update to matrix elements with/without shadow vectors
void print_instance_struct_copy_to_device() override
call helper function for copying the instance struct to the device
void print_rhs_d_shadow_variables() override
setup method for setting matrix shadow vectors
Visitor for printing C++ code with OpenACC backend
void print_dt_update_to_device() const override
update dt from host to device
void print_global_variable_device_update_annotation() override
update global variable from host to the device
void print_kernel_data_present_annotation_block_end() override
end of annotation like "acc enter data"
BlockType
Helper to represent various block types.
void print_abort_routine() const override
abort routine
void print_backend_includes() override
common includes : standard c++, coreneuron and backend specific
void print_instance_struct_delete_from_device() override
call helper function that deletes the instance struct from the device
void print_net_send_buf_update_to_host() const override
update NetSendBuffer_t from device to host
void print_net_send_buf_count_update_to_device() const override
update NetSendBuffer_t count from host to device
void print_instance_struct_transfer_routines(const std::vector< std::string > &ptr_members) override
define helper functions for copying the instance struct to the device
void print_net_init_acc_serial_annotation_block_end() override
end of annotation "acc kernels" for net_init kernel
std::string backend_name() const override
name of the code generation backend
void print_memory_allocation_routine() const override
memory allocation routine