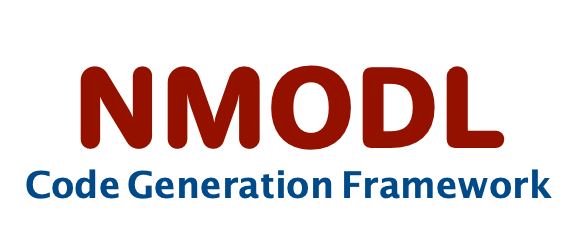 |
User Guide
|
Go to the documentation of this file.
24 #include <string_view>
41 using printer::CodePrinter;
288 std::vector<std::string>
const& ) {}
323 const std::string& name,
324 const std::unordered_set<CppObjectSpecifier>& specifiers = {
415 const std::
string& concentration) override;
508 const std::
string& name,
509 bool use_instance) const override;
520 bool use_instance = true) const override;
531 std::
string get_variable_name(const std::
string& name,
bool use_instance = true) const override;
638 const std::
string& function_name = "") override;
void print_coreneuron_includes()
Print includes from coreneuron.
bool needs_v_unused() const override
virtual std::string net_receive_buffering_declaration()
Generate the target backend code for the net_receive_buffering function delcaration.
virtual bool nrn_cur_reduction_loop_required()
Check if reduction block in nrn_cur required.
void print_net_move_call(const ast::FunctionCall &node) override
Print call to net_move.
void print_mechanism_range_var_structure(bool print_initializers) override
Print the structure that wraps all range and int variables required for the NMODL.
Represents a C code block.
virtual void print_net_send_buf_count_update_to_host() const
Print the code to update NetSendBuffer_t count from device to host.
Visitor for printing C++ code compatible with legacy api of CoreNEURON
void print_function_prototypes() override
Print function and procedures prototype declaration.
std::string process_verbatim_text(std::string const &text)
Process a verbatim block for possible variable renaming.
void print_v_unused() const override
Set v_unused (voltage) for NRN_PRCELLSTATE feature.
void print_derivimplicit_kernel(const ast::Block &block)
Print derivative kernel when derivimplicit method is used.
void visit_protect_statement(const ast::ProtectStatement &node) override
visit node of type ast::ProtectStatement
virtual void print_device_stream_wait() const
Print the code to synchronise/wait on stream specific to NrnThread.
virtual void print_instance_struct_delete_from_device()
Delete the instance struct from the device.
virtual void print_net_send_buf_count_update_to_device() const
Print the code to update NetSendBuffer_t count from host to device.
codegen::CodegenInfo info
All ast information for code generation.
std::string global_variable_name(const SymbolType &symbol, bool use_instance=true) const override
Determine the variable name for a global variable given its symbol.
virtual void print_newtonspace_transfer_to_device() const
Print code block to transfer newtonspace structure to device.
int thread_data_index
thread_data_index indicates number of threads being allocated.
void print_net_send_call(const ast::FunctionCall &node) override
Print call to net_send.
virtual void print_net_receive_loop_begin()
Print the code for the main net_receive loop.
virtual void print_kernel_data_present_annotation_block_begin()
Print accelerator annotations indicating data presence on device.
void print_g_unused() const override
Set g_unused (conductance) for NRN_PRCELLSTATE feature.
void print_common_getters()
Print common getters.
std::string int_variable_name(const IndexVariableInfo &symbol, const std::string &name, bool use_instance) const override
Determine the name of an int variable given its symbol.
void print_initial_block(const ast::InitialBlock *node)
Print initial block statements.
void print_net_receive_kernel()
Print net_receive kernel function definition.
void print_thread_getters()
Print the getter method for thread variables and ids.
std::string simulator_name() override
Name of the simulator the code was generated for.
void print_net_send_buffering()
Print kernel for buffering net_send events.
virtual std::string namespace_name() override
Name of "our" namespace.
encapsulates code generation backend implementations
int num_thread_objects() const noexcept
Determine the number of threads to allocate.
void print_fast_imem_calculation() override
Print fast membrane current calculation code.
virtual void print_dt_update_to_device() const
Print the code to update dt from host to device.
virtual void print_net_init_acc_serial_annotation_block_end()
Print accelerator kernels end annotation for net_init kernel.
const ParamVector external_method_parameters(bool table=false) noexcept override
Parameters for functions in generated code that are called back from external code.
void print_net_init()
Print initial block in the net receive block.
void print_net_event_call(const ast::FunctionCall &node) override
Print call to net_event.
void print_nrn_destructor() override
Print nrn_destructor function definition.
void print_sdlists_init(bool print_initializers) override
Implement classes for representing symbol table at block and file scope.
void print_headers_include() override
Print all includes.
void print_send_event_move()
Print send event move block used in net receive as well as watch.
void append_conc_write_statements(std::vector< ShadowUseStatement > &statements, const Ion &ion, const std::string &concentration) override
Generate Function call statement for nrn_wrote_conc.
virtual void print_instance_struct_copy_to_device()
Transfer the instance struct to the device.
void print_global_variables_for_hoc() override
Print byte arrays that register scalar and vector variables for hoc interface.
void print_net_receive_common_code(const ast::Block &node, bool need_mech_inst=true)
Print the common code section for net receive related methods.
void print_nrn_current(const ast::BreakpointBlock &node) override
Print the nrn_current kernel.
void print_function_table_call(const ast::FunctionCall &node) override
Print special code when calling FUNCTION_TABLEs.
void print_first_pointer_var_index_getter()
Print the getter method for index position of first pointer variable.
void print_setup_range_variable()
Print the function that initialize range variable with different data type.
std::string internal_method_arguments() override
Arguments for functions that are defined and used internally.
virtual void print_instance_struct_transfer_routine_declarations()
Print declarations of the functions used by print_instance_struct_copy_to_device and print_instance_s...
std::string nrn_thread_arguments() const override
Arguments for "_threadargs_" macro in neuron implementation.
void print_net_receive_arg_size_getter()
Print the getter method for getting number of arguments for net_receive.
std::pair< ParamVector, ParamVector > function_table_parameters(const ast::FunctionTableBlock &node) override
Parameters of the function itself "{}" and "table_{}".
void print_function_or_procedure(const ast::Block &node, const std::string &name, const std::unordered_set< CppObjectSpecifier > &specifiers={ CppObjectSpecifier::Inline}) override
Print nmodl function or procedure (common code)
Visitor for printing C++ code compatible with legacy api of CoreNEURON
void visit_verbatim(const ast::Verbatim &node) override
visit node of type ast::Verbatim
Base class for all block scoped nodes.
Represents a INITIAL block in the NMODL.
virtual void print_nrn_cur_matrix_shadow_update()
Print the update to matrix elements with/without shadow vectors.
std::string register_mechanism_arguments() const override
Arguments for register_mech or point_register_mech function.
virtual void print_net_init_acc_serial_annotation_block_begin()
Print accelerator kernels begin annotation for net_init kernel.
virtual void print_deriv_advance_flag_transfer_to_device() const
Print the code to copy derivative advance flag to device.
void print_first_random_var_index_getter()
Print the getter method for index position of first RANDOM variable.
Represent WATCH statement in NMODL.
virtual void print_before_after_block(const ast::Block *node, size_t block_id)
Print NMODL before / after block in target backend code.
Represents a BREAKPOINT block in NMODL.
void print_ion_variable() override
Print the ion variable struct.
Helper class for printing C/C++ code.
void print_ion_var_structure()
Print structure of ion variables used for local copies.
ParamVector internal_method_parameters() override
Parameters for internally defined functions.
int position_of_int_var(const std::string &name) const override
Determine the position in the data array for a given int variable.
virtual bool is_constant_variable(const std::string &name) const
Check if variable is qualified as constant.
void print_function_procedure_helper(const ast::Block &node) override
Common helper function to help printing function or procedure blocks.
void visit_for_netcon(const ast::ForNetcon &node) override
visit node of type ast::ForNetcon
void print_compute_functions() override
Print all compute functions for every backend.
void print_nrn_cur_conductance_kernel(const ast::BreakpointBlock &node) override
Print the nrn_cur kernel with NMODL conductance keyword provisions.
virtual void print_memory_allocation_routine() const
Print memory allocation routine.
void print_check_table_thread_function()
Print check_table functions.
std::string backend_name() const override
Name of the code generation backend.
virtual void print_rhs_d_shadow_variables()
Print the setup method for setting matrix shadow vectors.
void print_net_receive()
Print net_receive function definition.
bool vectorize
true if mod file is vectorizable (which should be always true for coreneuron) But there are some bloc...
bool optimize_ion_variable_copies() const override
Check if ion variable copies should be avoided.
Various types to store code generation specific information.
void print_mechanism_global_var_structure(bool print_initializers) override
Print the structure that wraps all global variables used in the NMODL.
void print_nrn_constructor() override
Print nrn_constructor function definition.
virtual void print_kernel_data_present_annotation_block_end()
Print matching block end of accelerator annotations for data presence on device.
virtual void print_instance_struct_transfer_routines(std::vector< std::string > const &)
Print the definitions of the functions used by print_instance_struct_copy_to_device and print_instanc...
void print_nrn_alloc() override
Print nrn_alloc function definition.
virtual void print_nrn_cur_matrix_shadow_reduction()
Print the reduction to matrix elements from shadow vectors.
void print_memb_list_getter()
Print the getter method for returning membrane list from NrnThread.
void print_watch_check()
Print watch activate function.
void print_instance_variable_setup()
Print the function that initialize instance structure.
void print_nrn_init(bool skip_init_check=true)
Print the nrn_init function definition.
std::string get_variable_name(const std::string &name, bool use_instance=true) const override
Determine variable name in the structure of mechanism properties.
virtual void print_abort_routine() const
Print backend specific abort routine.
void print_nrn_cur() override
Print nrn_cur / current update function definition.
std::string get_range_var_float_type(const SymbolType &symbol)
Returns floating point type for given range variable symbol.
virtual void print_net_send_buffering_cnt_update() const
Print the code related to the update of NetSendBuffer_t cnt.
void print_thread_memory_callbacks()
Print thread related memory allocation and deallocation callbacks.
Represent a callback to NEURON's derivimplicit solver.
void print_net_receive_buffering(bool need_mech_inst=true)
Print kernel for buffering net_receive events.
virtual void print_global_function_common_code(BlockType type, const std::string &function_name="") override
Print common code for global functions like nrn_init, nrn_cur and nrn_state.
ParamVector functor_params() override
The parameters of the Newton solver "functor".
Implement logger based on spdlog library.
parser::NmodlParser::symbol_type SymbolType
BlockType
Helper to represent various block types.
void print_mechanism_register() override
Print the mechanism registration function.
virtual void print_global_method_annotation()
Print backend specific global method annotation.
void print_nrn_cur_kernel(const ast::BreakpointBlock &node) override
Print main body of nrn_cur function.
std::string nrn_thread_internal_arguments() override
Arguments for "_threadargs_" macro in neuron implementation.
virtual void print_device_atomic_capture_annotation() const
Print pragma annotation for increase and capture of variable in automatic way.
void visit_derivimplicit_callback(const ast::DerivimplicitCallback &node) override
visit node of type ast::DerivimplicitCallback
Visitor for printing C++ code compatible with legacy api of CoreNEURON
CodegenCppVisitor(std::string mod_filename, std::ostream &stream, std::string float_type, const bool optimize_ionvar_copies, std::unique_ptr< nmodl::utils::Blame > blame=nullptr)
Constructs the C++ code generator visitor.
virtual void print_get_memb_list()
Print the target backend code for defining and checking a local Memb_list variable.
void print_num_variable_getter()
Print the getter methods for float and integer variables count.
void print_standard_includes() override
Print standard C/C++ includes.
virtual void print_net_receive_loop_end()
Print the code for closing the main net_receive loop.
void print_mech_type_getter()
Print the getter method for returning mechtype.
std::string float_variable_name(const SymbolType &symbol, bool use_instance) const override
Determine the name of a float variable given its symbol.
virtual void print_global_variable_device_update_annotation()
Print the pragma annotation to update global variables from host to the device.
void add_variable_tqitem(std::vector< IndexVariableInfo > &variables) override
Add the variable tqitem during get_int_variables.
void visit_watch_statement(const ast::WatchStatement &node) override
visit node of type ast::WatchStatement
const std::string external_method_arguments() noexcept override
Arguments for external functions called from generated code.
void print_nrn_cur_non_conductance_kernel() override
Print the nrn_cur kernel without NMODL conductance keyword provisions.
virtual void print_backend_includes()
Print backend specific includes (none needed for C++ backend)
std::string process_verbatim_token(const std::string &token)
Process a token in a verbatim block for possible variable renaming.
void print_nrn_state() override
Print nrn_state / state update function definition.
void add_variable_point_process(std::vector< IndexVariableInfo > &variables) override
Add the variable point_process during get_int_variables.
void print_codegen_routines() override
Print entry point to code generation.
void print_watch_activate()
Print watch activate function.
virtual void print_atomic_reduction_pragma()
Print atomic update pragma for reduction statements.
virtual void print_net_send_buffering_grow()
Print statement that grows NetSendBuffering_t structure if needed.
virtual void print_net_send_buf_update_to_host() const
Print the code to update NetSendBuffer_t from device to host.
void print_data_structures(bool print_initializers) override
Print all classes.
std::string replace_if_verbatim_variable(std::string name)
Replace commonly used verbatim variables.
virtual void print_ion_var_constructor(const std::vector< std::string > &members)
Print constructor of ion variables.
int position_of_float_var(const std::string &name) const override
Determine the position in the data array for a given float variable.
std::vector< std::tuple< std::string, std::string, std::string, std::string > > ParamVector
A vector of parameters represented by a 4-tuple of strings:
Concrete visitor for all AST classes.